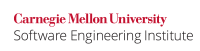
...
The DiaryPool
class creates a thread pool that reuses a fixed number of threads operating off a shared, unbounded queue. At any point, at most, no more than numOfThreads
threads are actively processing tasks. If additional tasks are submitted when all threads are active, they wait in the queue until a thread is available. The thread-local state of the thread persists when a thread is recycled.
...
Although increasing the size of the thread pool resolves the problem for this example, it fails to scale because changing the thread pool size is insufficient when if additional tasks can be submitted to the pool.
...
Code Block | ||
---|---|---|
| ||
public final class Diary { // ... public static void removeDay() { days.remove(); } } public final class DiaryPool { // ... public void doSomething1() { exec.execute(new Runnable() { @Override public void run() { try { Diary.setDay(Day.FRIDAY); diary.threadSpecificTask(); } finally { Diary.removeDay(); // Diary.setDay(Day.MONDAY) // can also be used } } }); } // ... } |
...
Code Block | ||
---|---|---|
| ||
class CustomThreadPoolExecutor extends ThreadPoolExecutor { public CustomThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue) { super(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue); } @Override public void beforeExecute(Thread t, Runnable r) { if (t == null || r == null) { throw new NullPointerException(); } Diary.setDay(Day.MONDAY); super.beforeExecute(t, r); } } public final class DiaryPool { // ... DiaryPool() { exec = new CustomThreadPoolExecutor(NumOfthreads, NumOfthreads, 10, TimeUnit.SECONDS, new ArrayBlockingQueue<Runnable>(10)); diary = new Diary(); } // ... } |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="6ef210cd5b940056-ff14ce24-455d44a8-9e90b8c5-326f442829a1688b49b5a3d2"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | class | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a10123ffaf7043b2-8f2ee547-4cf7457d-a74eb721-594d3113a832e421ccad26fe"><ac:plain-text-body><![CDATA[ | [[JPL 2006 | AA. Bibliography#JPL 06]] | 14.13, | ]]></ac:plain-text-body></ac:structured-macro> |
...