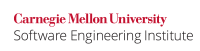
...
This compliant solution eliminates the null
return and simply returns the List
, even if it is zero-length. The client can effectively handle this situation without being interrupted by runtime exceptions. When arrays are returned instead of collections, care must be taken to ensure that the client does not access individual elements of a zero-length array. This prevents an ArrayOutOfBoundsException
.
Code Block | ||
---|---|---|
| ||
class Inventory { private final Hashtable<String, Integer> items; public Inventory() { items = new Hashtable<String, Integer>(); } public List<String> getStock() { List<String> l = new ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((noOfItems = items.get(value)) == 0) { l.add((String)value); } } return l; // Return list (possibly zero-length) } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); List<String> items = iv.getStock(); System.out.println(items.size()); // Does not throw a NPE } } |
...