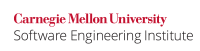
...
In this noncompliant code example, MutableClass
uses a mutable field date
of type Date
. Class Date
is also a mutable class. The example is noncompliant because the MutableClass
objects lack copy functionality.
Code Block | ||
---|---|---|
| ||
public final class MutableClass {
private Date date;
public MutableClass(Date d) {
this.date = d;
}
public void setDate(Date d) {
this.date = d;
}
public Date getDate() {
return date;
}
}
|
...
This compliant solution uses a copy constructor that initializes a MutableClass
instance when an argument of the same type (or subtype) is passed to it.
Code Block | ||
---|---|---|
| ||
public final class MutableClass { // Copy Constructor
private final Date date;
public MutableClass(MutableClass mc) {
this.date = new Date(mc.date.getTime());
}
public MutableClass(Date d) {
this.date = new Date(d.getTime()); // Make defensive copy
}
public Date getDate() {
return (Date) date.clone(); // Copy and return
}
}
|
...
This compliant solution exports a public static factory method getInstance()
that creates and returns a copy of a given MutableClass
object instance.
Code Block | ||
---|---|---|
| ||
class MutableClass {
private final Date date;
private MutableClass(Date d) { // Noninstantiable and nonsubclassable
this.date = new Date(d.getTime()); // Make defensive copy
}
public Date getDate() {
return (Date) date.clone(); // Copy and return
}
public static MutableClass getInstance(MutableClass mc) {
return new MutableClass(mc.getDate());
}
}
|
...
This compliant solution provides the needed copy functionality by declaring MutableClass
to be final, implementing the Cloneable
interface, and providing an Object.clone()
method that performs a deep copy of the object.
Code Block | ||
---|---|---|
| ||
public final class MutableClass implements Cloneable {
private Date date;
public MutableClass(Date d) {
this.date = new Date(d.getTime());
}
public Date getDate() {
return (Date) date.clone();
}
public void setDate(Date d) {
this.date = (Date) d.clone();
}
public Object clone() throws CloneNotSupportedException {
final MutableClass cloned = (MutableClass) super.clone();
cloned.date = (Date) date.clone(); // manually copy mutable Date object
return cloned;
}
}
|
...
When a mutable class's instance fields are declared final and lack accessible copy methods, provide a clone()
method, as shown in this compliant solution.
Code Block | ||
---|---|---|
| ||
public final class MutableClass implements Cloneable {
private final Date date; // final field
public MutableClass(Date d) {
this.date = new Date(d.getTime()); // copy-in
}
public Date getDate() {
return (Date) date.clone(); // copy and return
}
public Object clone() {
Date d = (Date) date.clone();
MutableClass cloned = new MutableClass(d);
return cloned;
}
}
|
...
If cloning or copying a mutable object is infeasible or expensive, one alternative is to create an unmodifiable view class. This class overrides mutable methods to throw an exception, protecting the mutable class.
Code Block | ||
---|---|---|
| ||
class UnmodifiableDateView extends Date {
private Date date;
public UnmodifiableDateView(Date date) {
this.date = date;
}
public void setTime(long date) {
throw new UnsupportedOperationException();
}
// Override all other mutator methods to throw UnsupportedOperationException
}
public final class MutableClass {
private Date date;
public MutableClass(Date d) {
this.date = d;
}
public void setDate(Date d) {
this.date = (Date) d.clone();
}
public UnmodifiableDateView getDate() {
return new UnmodifiableDateView(date);
}
}
|
...
Sound automated detection is infeasible in the general case. Heuristic approaches could be useful.
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | FB.EI_EXPOSE_REP2 FB.EI_EXPOSE_REP | Implemented |
Related Guidelines
CWE-374. Passing Mutable Objects to an Untrusted Method | |
| CWE-375. Returning a Mutable Object to an Untrusted Caller |
Secure Coding Guidelines for the Java Programming Language, Version 3.0 | Guideline 2-3. Support copy functionality for a mutable class |
...
[API 2006] | |
Item 39. Make defensive copies when needed, Item 11. Override clone judiciously | |
04. Object Orientation (OBJ) OBJ05-J. Defensively copy private mutable class members before returning their references