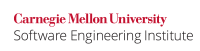
Applying a lock over a call to a method performing network transactions can be problematic. Depending on the speed and reliability of the connection, the synchronization could lock up can stall the program , producing indefinitely causing a huge performance hit. At other times, it can result in temporary or permanent deadlock.
...
This noncompliant code example involves the method send_page
sendPage()
which sends a Page
object containing information being passed between a client and server. send_packet
The method is synchronized to protect access to the array page_buff
pageBuff
. Calling writeObject
within the synchronized sendPage
can lead to a deadlock for high latency or lossy network connections.
Code Block | ||
---|---|---|
| ||
// Class Page is defined separately. It stores and returns the Page name via getName() public final boolean SUCCESS = true; public final boolean FAILURE = false; Page[] pageBuff = new Page[MAX_PAGE_SIZE]; public synchronized boolean send_pagesendPage(Socket socket, String page_namepageName){ try{ // Get the output stream to write the Page to ObjectOutputStream outStreamout = new ObjectOutputStream(socket.getOutputStream()); Page target_pagetargetPage = null; // Find the Page requested by the client for(Page p : page_buffpageBuff){ if(p.getName().equalscompareTo(page_name)pageName) == 0) target_pagetargetPage = p; } // Page requested does not exist if(target_pagetargetPage == null) return FAILURE; // Send the Page to the client out.writeObject(target_pagetargetPage); out.flush(); return SUCCESSout.close(); } catch(IOException io){ /* handle exception /*/ handle} exception return SUCCESS; } } |
...
Compliant Solution
A solution would be to separate One compliant solution entails separating the actions into a sequence of steps:
- Perform actions on data structures requiring synchronization.
- If sending data, create Create copies of Objects to send.
- Perform network calls in a separate method.
In the following example, the synchronized method get_page
getPage()
is called from SendReply
to find the appropriate Page
requested by the client from the Page
array page_buff
. It then pageBuff
. The method sendReply()
in turn calls the unsynchronized method send_page
(which has no synchronization applied to it sendPage()
to send deliver the Page
.
Code Block | ||
---|---|---|
| ||
public final boolean SUCCESS = true; public final boolean FAILURE = false; public boolean send_replysendReply(Socket socket, String page_namepageName){ Page target_pagetargetPage = get_page(page_namegetPage(pageName); if(target_pagetargetPage == null) return FAILURE; return send_pagesendPage(Socket socket, target_pagetargetPage); } private synchronized Page get_pagegetPage(String page_namepageName){ Page target_pagetargetPage = null; for(Page p : page_buffpageBuff){ if(p.getName().equals(page_namepageName)) target_pagetargetPage = p; } return target_pagetargetPage; } public boolean send_pagesendPage(Socket socket, Page page){ try{ // Get the output stream to write the Page to ObjectOutputStream outStreamout = new ObjectOutputStream(socket.getOutputStream()); // Send the Page to the client out.writeObject(page); out.flush(); out.close(); return SUCCESS; }catch(IOException io){ // handle exception else return failure return FAILURE; } } |
General recommendations for correct use of locks and synchronization appear in CON00-J. Use synchronization judiciously.
Risk Assessment
Application of synchronization or locks to methods that perform transactions over a network can lead to If monitor regions such as synchronized methods and statements contain network transactional logic, temporary or permanent deadlocks may result.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON37-J | low | likely | high | P3 | L3 |
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.General recommendations for correct use of locks and synchronization appear in CON00-J. Use synchronization judiciously.
References
Wiki Markup |
---|
\[[Grosso 01|AA. Java References#Grosso 01]\] [Chapter 10: Serialization|http://oreilly.com/catalog/javarmi/chapter/ch10.html] \[[JLS 05|AA. Java References#JLS 05]\] [Chapter 17, Threads and Locks|http://java.sun.com/docs/books/jls/third_edition/html/memory.html] \[[Rotem 08|AA. Java References#Rotem 08]\] [Falacies of Distributed Computing Explained|http://www.rgoarchitects.com/Files/fallacies.pdf] |
...