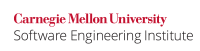
...
Code Block | ||
---|---|---|
| ||
import java.io.BufferedInputStream; import java.io.EOFException; public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); int input = in.read(); if (input == -1) { throw new EOFException(); } return (char)input; //okay because InputStream guarantees read() fits in a byterange 0..255 if it is not -1 } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } } |
...
Code Block | ||
---|---|---|
| ||
import java.io.BufferedInputStream; import java.io.EOFException; public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); //This statement now necessary to go to the next line //the Noncompliant code example deceptively worked without it return (char)input; //okay because InputStream guarantees read() fits in a byterange 0..255 if it is not -1 } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } } |
...