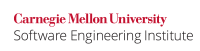
A switch statement is comprised of several case labels, and a default label. The default label is not required, but strongly recommended. The statements following a case label conventionally end with a break; statement, which moves control flow to the end of the switch block. If omitted, control flow falls through to the next case statement in the switch block. Because the break statement is not required, omitting it produces no compiler warnings, and if this was unintentional, it can lead to an unexpected control flow.
Noncompliant Code Example
In this noncompliant code example, the case where card = 11 does not have a break statement. Thus, the statements for card = 12 are also executed when card = 11.
Code Block | ||
---|---|---|
| ||
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; } |
Compliant Solution
In the compliant solution, each case label is ended with a break statement.
Code Block | ||
---|---|---|
| ||
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); break; case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; } |
Exceptions
EX1: The last label in a switch statement requires no break. The break statement serves to skip to the end of the switch block, so control flow will continue to statements following the switch block with or without it. . Conventionally, the last label is the default label.
EX2: In some cases, where control flow is intended to execute the same code for multiple cases, it is permissible to omit the break statement. However, these instances must be explicitly documented.
Code Block | ||
---|---|---|
| ||
int card=11; int value; /* Case 11,12,13 fall through to the same case */ switch (card) { /* ... */ case 11: case 12: case 13: value=10; break; default: /* Handle Error Condition */ } |
Risk Assessment
Failure to include break statements leads to unexpected control flow.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
| medium | likely | low | P6 | L2 |
Automated Detection
Unknown
Other Languages
This rule appears in the C++ Secure Coding Standard as MSC18-CPP. Finish every set of statements associated with a case label with a break statement and MSC17-C. Finish every set of statements associated with a case label with a break statement.