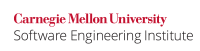
...
Code Block | ||
---|---|---|
| ||
import java.util.regex.Pattern; import java.util.regex.Matcher; import java.util.HashMap; /\* Usage Test2 <regex> \* Regex is used directly without santization causing sensitive data to be exposed \* \* Imagine this program searches a database of users for usernames that match a regex \* Non malicious usage: Test1 John.\* \* Malicious usage: (?s)John.\* */ public class Test2 { public static class User { String name, password; public User(String name, String password) { Â Â Â Â Â Â Â Â Â setName(name); Â Â Â Â Â Â Â Â Â setPassword(password); Â Â Â Â Â Â } private void setName(String n) { name = n; } private void setPassword(String pw) { password = pw; } public String getName() { return name; } } public static void main(String\[\] args) { if (args.length < 1) { Â Â Â Â Â Â Â Â Â System.err.println("Failed to specify a regex"); Â Â Â Â Â Â Â Â Â return; Â Â Â Â Â Â } String sensitiveData; //represents sensitive data from a file or something int flags; String regex; Pattern p; Matcher m; HashMap<String, User> userMap = new HashMap<String, User>(); //imagine a CSV style database: user,password sensitiveData = "JohnPaul,HearsGodsVoice\nJohnJackson,OlympicBobsleder\nJohnMayer,MakesBadMusic\n"; String\[\] csvUsers = sensitiveData.split("\n"); for (String csvUser : csvUsers) { Â Â Â Â Â Â Â Â Â String[] csvUserSplit = csvUser.split(","); Â Â Â Â Â Â Â Â Â String name = csvUserSplit[0]; Â Â Â Â Â Â Â Â Â String pw = csvUserSplit[1]; Â Â Â Â Â Â Â Â Â User u = new User(name, pw); Â Â Â Â Â Â Â Â Â userMap.put(name, u); Â Â Â Â Â Â } regex = args[0]; flags = 0; System.out.println("Pattern: \'" + regex + "\'"); p = Pattern.compile(regex, flags); for (String u : userMap.keySet()) { Â Â Â Â Â Â Â Â Â m = p.matcher(u); Â Â Â Â Â Â Â Â Â while (m.find()) Â Â Â Â Â Â Â Â Â Â Â Â System.out.println("Found \'" + m.group() + "\'"); Â Â Â Â Â Â } System.err.println("DONE"); } } | ||
Code Block |
Risk Assessment
Rule | Severity | Liklihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
IDS18-J | medium | unlikely | high |
|
|
...
CWE ID 625 Permissive Regular Expressions
\[CVE-2005-1949\|[http://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2005-1949|http://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2005-1949]\] Arbitrary command execution in ePing plugin for e107 portal due to an overly permissive regular expression parsing an IP Wiki Markup