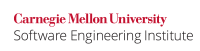
...
Code Block | ||
---|---|---|
| ||
final class BankAccount implements Comparable<BankAccount> { private double balanceAmount; // Total amount in bank account private final Object lock; private final long id; // Unique for each BankAccount private static long nextID = 0; // Next unused id private BankAccount(double balance) { this.balanceAmount = balance; this.lock = new Object(); this.id = this.nextID++; } public int compareTo(BankAccount ba) { if (this.id < ba.id) { return -1; }// No need to check for overflow because the type long is large if (this.id > ba.id) { return 1;// enough to hold the required number of bank accounts } return 0;return (this.id - ba.id); } // Deposits the amount from this object instance to BankAccount instance argument ba public void depositAmount(BankAccount ba, double amount) { BankAccount former, latter; if (compareTo(ba) < 0) { former = this; latter = ba; } else { former = ba; latter = this; } synchronized (former) { synchronized (latter) { if (amount > balanceAmount) { throw new IllegalArgumentException("Transfer cannot be completed"); } ba.balanceAmount += amount; this.balanceAmount -= amount; } } } public static void initiateTransfer(final BankAccount first, final BankAccount second, final double amount) { Thread t = new Thread(new Runnable() { public void run() { first.depositAmount(second, amount); } }); t.start(); } } |
...