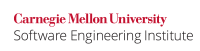
...
In this noncompliant example, MutableClass
uses a mutable Date
object. If the caller changes the instance of the Date
object (like incrementing the month), the class implementation no longer remains consistent with its old state. Both, the constructor as well as the getDate
method are susceptible to abuse. This also defies attempts to implement thread safety.
Code Block | ||
---|---|---|
| ||
import java.util.Date; public final class MutableClass { private Date d; public MutableClass(Date d) { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } this.d = d; } public Date getDate() { return this.d; } } |
This code also violates OBJ37-J. Do not return references to private data
...
Always provide mechanisms to create copies of the instances of a mutable class. This compliant solution implements the Cloneable
interface and overrides the clone
method to create a deep copy of both the object and the mutable Date
object. Since using clone()
independently only produces a shallow copy and still leaves the class mutable, it is advised to also copy all the referenced mutable objects that are passed in or returned from any method.
Code Block | ||
---|---|---|
| ||
import java.util.Date; public final class CloneClass implements Cloneable { private Date d; public CloneClass(Date d) { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } this.d = new Date(d.getTime()); //copy-in } public Object clone() throws CloneNotSupportedException { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } final CloneClass cloned = (CloneClass)super.clone(); cloned.d = (Date)this.d.clone(); //copy mutable Date object manually return cloned; } public Date getDate() { return new Date(this.(Date)d.getTimeclone()); //copy and return } } |
At times, a class is labeled final
with no accessible copy methods. Callers can then obtain an instance of the class, create a new instance with the original state and subsequently proceed to use it. Similarly, mutable objects obtained must also be copied when necessary.
...