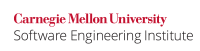
...
Code Block | ||
---|---|---|
| ||
import java.util.Date; public class ExceptionExample { public static void untrustedCode() { Date now = new Date(); Class<?> dateClass = now.getClass(); createInstance(dateClass); } public static void createInstance(Class<?> dateClass) { try { // Create another Date object using the Date Class Object o = dateClass.newInstance(); if (o instanceof Date) { Date d = (Date)o; System.out.println("The time is: " + d.toString()); } } catch (InstantiationException ie) { System.out.println(ie.toString()); } catch (IllegalAccessException iae) { System.out.println(iae.toString()); } } } |
A related issue is described in SEC04-J. Beware of standard APIs that perform access checks against the immediate caller.
Compliant Solution
Do not accept Class
, ClassLoader
or Thread
instances from untrusted code. If inevitable, safely acquire these instances by ensuring they come from trusted sources. Additionally, make sure to discard tainted inputs from untrusted code. Likewise, objects returned by the affected methods should not be propagated back to the untrusted code.
...