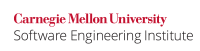
...
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { long temp = (long)a + (long)b; if(temp >Integer.MAX_VALUE || temp < Integer.MIN_VALUE) { throw new ArithmeticException(); } return (int)temp; // Value within range can perform the addition } |
...
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { if( (b>0 && (a > Integer.MAX_VALUE - b)) || (b<0 && (a < Integer.MIN_VALUE - b)) ) { throw new ArithmeticException(); } return a + b; //Value within range can perform the addition } |
...
Code Block | ||
---|---|---|
| ||
public boolean overflow(long a, long b) { BigInteger ba = new java.math.BigInteger(String.valueOf(a)); BigInteger bb = new java.math.BigInteger(String.valueOf(b)); BigInteger br = ba.add(bb); return (br.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) == 1 || br.compareTo(BigInteger.valueOf(Long.MIN_VALUE))== -1); } public long do_operation(long a, long b) throws ArithmeticException { if(overflow(a,b)) { throw new ArithmeticException(); } // Within range; safely perform the addition return a + b; } |
...
Code Block | ||
---|---|---|
| ||
public int do_operation(int a,int b) { long temp = (long)a - (long)b; if(temp < Integer.MIN_VALUE || temp > Integer.MAX_VALUE) { throw new ArithmeticException(); } return (int) temp; } |
Compliant Solution (Bounds Checking)
...
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { if( (b>0 && (a < Integer.MIN_VALUE + b)) || (b<0 && (a > Integer.MAX_VALUE + b)) ) { throw new ArithmeticException(); } return a - b; //Value within range can perform the addition } |
...
Code Block | ||
---|---|---|
| ||
public boolean underflow(long a, long b) { BigInteger ba = new BigInteger(String.valueOf(a)); BigInteger bb = new BigInteger(String.valueOf(b)); BigInteger br = ba.subtract(bb); return (br.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) == 1 || br.compareTo(BigInteger.valueOf(Long.MIN_VALUE)) == -1); } public long do_operation(long a, long b) throws ArithmeticException { if(underflow(a,b)) { throw new ArithmeticException(); } // Within range; safely perform the subtraction return a - b; } |
...
Code Block | ||
---|---|---|
| ||
int a,b,result; long temp = (long) a * (long)b; if(temp > Integer.MAX_VALUE || temp < Integer.MIN_VALUE) { throw new ArithmeticException(); // Overflow } result = (int) temp; // Value within range, safe to downcast |
...
Code Block | ||
---|---|---|
| ||
if(a == Integer.MIN_VALUE && b == -1) { throw new ArithmeticException(); // May be Integer.MIN_VALUE and -1 } result = a/b; // Safe operation |
...
Code Block | ||
---|---|---|
| ||
if(result == Integer.MIN_VALUE) { throw new ArithmeticException(); } temp = -result; |
Absolute Value
...