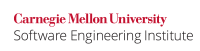
The Thread-Per-Message design is the simplest concurrency technique wherein a thread is created for each incoming request. The benefits of creating a new thread to handle each request should outweigh the corresponding thread creation overheads. This design strategy is generally recommended over sequential executions for time consuming, I/O bound, session based or isolated tasks.
Wiki Markup |
---|
On the other hand, there can be several disadvantagespitfalls of this design strategy such as thread creation overhead in case of frequent or recurring requests, significant processing overhead, resource exhaustion of threads (leading to {{OutOfMemoryError}}), thread scheduling and context switching overhead \[[Lea 00|AA. Java References#Lea 00]\]. |
An Furthermore, an attacker can cause a denial of service by overwhelming the system with too many requests, all at once. Instead of degrading gracefully, the system goes down becomes unresponsive abruptly, resulting in an availability issue. Thread pools allow the system to service as many requests as it can comfortably sustain, instead of stopping terminating all services when faced with a deluge of requests. From the safety point of view, it is possible for one component to exhaust all resources because of some intermittent error, starving all others from using them.
Thread Pools overcome these issues as because the maximum number of worker threads that can be initiated and executed simultaneously concurrently can be suitably controlled. Every worker accepts a Runnable
object from a request and stores it in a temporary Channel
like a buffer or a queue until resources become available. Because threads are reused and can be efficiently added to the Channel
, most of the thread creation overhead is also eliminated.
...
This noncompliant code example demonstrates the Thread-Per-Message design that fails to provide graceful degradation of service. The class RequestHandler
provides a public static factory method so that callers can obtain an its instance. Subsequently, the handleRequest()
method can be is used to handle each request in its own thread.
Code Block | ||
---|---|---|
| ||
class Helper {
public void handle(Socket socket) {
//...
}
}
final class RequestHandler {
private final Helper helper = new Helper();
private final ServerSocket server;
private RequestHandler(int port) throws IOException {
server = new ServerSocket(port);
}
public static RequestHandler getInstance(int port) throws IOException {
return new RequestHandler(port);
}
public void handleRequest() {
new Thread(new Runnable() {
public void run() {
try {
helper.handle(server.accept());
} catch (IOException e) {
// Forward to handler
}
}
}).start();
}
// Other methods such as for shutting down the thread pool and task cancellation ...
}
|
Compliant Solution
Wiki Markup |
---|
This compliant solution uses a _Fixed Thread Pool_ that places an upper bound on the number of concurrently executing threads. Tasks submitted to the pool are stored in an internal queue. This prevents the system from being overwhelmed when trying to respond to all incoming requests and allows it to degrade gracefully by serving a fixed number of clients at a particular time. \[[Tutorials 08|AA. Java References#Tutorials 08]\] |
...
The choice of the unbounded newFixedThreadPool
may not always be the best. Refer to the API documentation for choosing between newFixedThreadPool
, newCachedThreadPool
, newSingleThreadExecutor
and newScheduledThreadPool
to meet the specific design requirements.
Risk Assessment
...