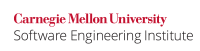
...
Code Block | ||
---|---|---|
| ||
import java.io.BufferedInputStream; import java.io.IOException; public final class InputLibrary{ public static char getChar()throws IOException { BufferedInputStream in = new BufferedInputStream(System.in); int input = in.read(); if(input==-1){ throw new IOException("Premature end of input."); } return (char)input; //okay because InputStream guarantees read() will fit in a byte if it is not -1 } public static void main(String[] args)throws IOException { System.out.print("Enter first initial: "); char first=getChar(); System.out.println("Your first initial is "+first); System.out.print("Enter last initial: "); char last=getChar(); System.out.println("Your last initial is "+last); } } |
...
Code Block | ||
---|---|---|
| ||
import java.io.BufferedInputStream; import java.io.IOException; public final class InputLibrary{ private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar()throws IOException { int input = in.read(); if(input==-1){ throw new IOException("Premature end of input."); } in.skip(1); //This line now necessary to go to the next line //in the previous example code deceptively worked without it return (char)input; //okay because InputStream guarantees read() will fit in a byte if it is not -1 } public static void main(String[] args)throws IOException { System.out.print("Enter first initial: "); char first=getChar(); System.out.println("Your first initial is "+first); System.out.print("Enter last initial: "); char last=getChar(); System.out.println("Your last initial is "+last); } } |
...