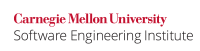
Do not operate on tainted inputs data in a doPrivileged()
block. An adversary may supply malicious input that might result in privilege escalation attacks.
...
This noncompliant code example accepts a tainted filename
argument. An adversary may supply the name pathname of a sensitive password file, complete with the path and consequently force operations to be performed on the wrong thus allowing an unprivileged user to access a protected file.
Code Block | ||
---|---|---|
| ||
private void privilegedMethod(final String filename) throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(filename); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
...
This compliant solution explicitly hardcodes the name of the file and confines the variables used in the privileged block to the same method. This ensures that no malicious file can be loaded by exploiting the privileges of the corresponding code.
Code Block | ||
---|---|---|
| ||
static final String FILEPATH = "/path/to/protected/file/fn.ext"; private void privilegedMethod() throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream("/usr/home/filename"FILEPATH); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
...
Risk Assessment
Allowing tainted inputs in privileged operations can lead to privilege escalation attacks.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC03-J | high | likely | low | P27 | L1 |
Automated Detection
Tools that support Taint Analysis enable code usage that is substantially similar to the Noncompliant Code Example. Typical taint analyses assume that there exists a method (or methods) that can "clean" potentially tainted inputs, providing untainted outputs (or appropriate errors, of course). The taint analysis then ensures that only untainted data is used inside the doPrivileged
block. Note that the static analyses necessarily assume that the cleaning methods are always successful; reality may vary.
Because the annotations used by the analysis tools vary, we present a notional example here.
Code Block | bgColor | #ccccff
---|
private void privilegedMethod(final String filename) throws FileNotFoundException { final String cleanFilename; try { cleanFilename = cleanAFilenameAndPath(filename); } catch (/* exception as per spec of cleanAFileNameAndPath */) { // log or forward to handler as appropriate based on specification // of cleanAFilenameAndPath } try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(cleanFilename); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
Risk Assessment
...
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC03-J | high | likely | low | P27 | L1 |
Automated Detection
Static taint analysis addresses part of this guideline, by tracking which data is known to be untainted. Useful taint analyses can also enforce rules such as "only untainted data inside doPrivileged
blocks.
All static taint analysis depends on the strength of the methods that clean potentially tainted data.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
...
Wiki Markup |
---|
\[[API 2006|AA. Bibliography#API 06]\] [method doPrivileged()|http://java.sun.com/javase/6/docs/api/java/security/AccessController.html#doPrivileged(java.security.PrivilegedAction)] \[[Gong 2003|AA. Bibliography#Gong 03]\] Sections 6.4, "AccessController" and 9.5 "Privileged Code" \[[Jovanovic 2006|AA. Bibliography#Jovanovic 06]\] "Pixy: A Static Analysis Tool for Detecting Web Application Vulnerabilities" \[[SCG 2007|AA. Bibliography#SCG 07]\] Guideline 6-1 "Safely invoke java.security.AccessController.doPrivileged" \[[MITRE 2009|AA. Bibliography#MITRE 09]\] [CWE ID 266|http://cwe.mitre.org/data/definitions/266.html] "Incorrect Privilege Assignment,", [CWE ID 272|http://cwe.mitre.org/data/definitions/272.html] "Least Privilege Violation" and [CWE ID 732|http://cwe.mitre.org/data/definitions/732.html] "Incorrect Permission Assignment for Critical Resource" |
...
SEC02-J. Guard doPrivileged blocks against untrusted invocation and leakage of sensitive data 02. Platform Security (SEC) SEC04-J. Do not expose standard APIs that may bypass Security Manager checks to untrusted code