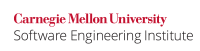
...
Programmers sometimes assume that using a thread-safe Collection
does not require explicit synchronization which is a misleading thought. It follows that using a thread-safe Collection
by itself does not ensure program correctness unless special care is taken to ensure that the client performs all related and independently atomic operations, as one atomic operation. For example, the standard thread-safe API may not provide a method to find a particular person's record in a Hashtable
and update the corresponding payroll information. In such cases, a custom thread-safe method must be designed and used. This guideline shows the need of such a method that performs a group of independently atomic operations as one atomic operation, and also suggests techniques for incorporating the method using a custom API.
This guideline applies to all uses of Collection
classes including the thread-safe Hashtable
class. Enumerations of the objects of a Collection
and iterators also require explicit synchronization on the Collection
object or any single lock object.
...
Code Block | ||
---|---|---|
| ||
class Helper {
public List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>());
public synchronized void addIPAddress(InetAddress ia) {
// Validate
ips.add(ia);
}
public synchronized void doSomething() throws UnknownHostException {
InetAddress[] ia;
ia = (InetAddress[]) ips.toArray(new InetAddress[0]);
System.out.println("Number of IPs: " + ia.length);
}
}
|
This noncompliant code example also violates OBJ00-J. Declare data members private.
Compliant Solution (Synchronized block)
...