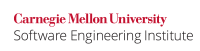
...
Code Block | ||
---|---|---|
| ||
class BankAccount {
private int balanceAmount; // Total amount in bank account
private final Lock lock = new ReentrantLock();
private static final int TIME = 1000; // 1 second
private BankAccount(int balance) {
this.balanceAmount = balance;
}
// Deposits the amount from this object instance to BankAccount instance argument ba
private void depositAllAmount(BankAccount ba) throws InterruptedException {
while (true) {
if (this.lock.tryLock()) {
try {
if (ba.lock.tryLock()) {
try {
ba.balanceAmount += this.balanceAmount;
this.balanceAmount = 0; // withdraw all amount from this instance
ba.displayAllAmount(); // Display the new balanceAmount in ba
break;
} finally {
ba.lock.unlock();
}
}
} finally {
this.lock.unlock();
}
}
Thread.sleep(TIME);
}
}
private void displayAllAmount() throws InterruptedException {
while (true) {
if (lock.tryLock()) {
try {
System.out.println(balanceAmount);
break;
} finally {
lock.unlock();
}
}
Thread.sleep(TIME);
}
}
public static void initiateTransfer(final BankAccount first, final BankAccount second) {
Thread t = new Thread(new Runnable() {
public void run() {
try {
first.depositAllAmount(second);
} catch (InterruptedException e) {
// Forward to handler
Thread.currentThread().interrupt(); // Reset interrupted status
}
}
});
t.start();
}
}
|
...