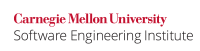
The write()
method defined in java.io.OutputStream
takes an integer argument intended to be between 0 and 255. Because an int
is otherwise designed to store 4 byte numbers, failure to range check may lead to unexpected resultstruncation of the higher order bits of the input.
Wiki Markup |
---|
The general contract for the {{write()}} method says that one byte is written to the output stream. The byte to be written constitutes the eight lower order bits of the argument {{b}}, passed to the {{write()}} method. The 24 high-order bits of {{b}} are ignored. \[[API 06|AA. Java References#API 06]\] |
...
Alternatively, perform range checking to be compliant. While this particular solution still does not display the original invalid integer
correctly, it behaves well when the corresponding read
method is used to convert the byte
back to an integer
. This is because it guarantees that the byte will contain representable data.
Code Block | ||
---|---|---|
| ||
class FileWrite { public static void main(String[] args) throws NumberFormatException, IOException { FileOutputStream out = new FileOutputStream("output.txt"); //Perform range checking if(Integer.valueOf(args[0]) >= 0 && Integer.valueOf(args[0]) <= 255) { out.write(Integer.valueOf(args[0].toString())); System.out.flush(); } else { //handle error throw new ArithmeticException("Value is out of range"); } } } |
...