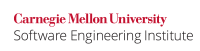
It is highly unlikely that a method is built to deal with all possible runtime exceptions; consequently no method should ever catch RuntimeException
. If a method catches RuntimeException
, it will may receive exceptions it was not designed to handle, such as NullPointerException
. Many catch
clauses simply log or ignore their error, and resume control flow. But runtime exceptions represent a bug execution resumes. Runtime exceptions indicate bugs in the program that should be fixed by the developer, and . They almost always lead to control flow vulnerabilities.
Likewise, a method should never catch Exception
or Throwable
, since because this implies catching RuntimeException
.
...
The following function takes a string String
and returns true
if it consists of a capital letter succeeded by lowercase letters. To handle corner cases, it merely wraps the code in a try
/-catch
block and reports any exceptions that arise.
Code Block | ||
---|---|---|
| ||
boolean isCapitalized(String s) { try { if (s.equals("")) { return true; } String first = s.substring( 0, 1); String rest = s.substring( 1); return (first.equals (first.toUpperCase()) && rest.equals (rest.toLowerCase())); } catch (RuntimeException exception) { ExceptionReporter.report (exception); } return false; } |
This code will report reports errors such as if s
is a null pointer, or is the an empty string String
. However, it will also catch unintentionally catches other errors that are unlikely to be handled properly, such as if the string belongs to a different thread.
Compliant Solution
Intead Instead of catching RuntimeException
, a program should catch very be as specific in catching exceptions as possible.
Code Block | ||
---|---|---|
| ||
boolean isCapitalized(String s) { try { if (s.equals("")) { return true; } String first = s.substring( 0, 1); String rest = s.substring( 1); return (first.equals (first.toUpperCase()) && rest.equals (rest.toLowerCase())); } catch (NullPointerException exception) { ExceptionReporter.report (exception); } return false; } |
This code will only catch catches those exceptions that are intended by the programmer to be caught. A For example, a concurrency - based exception will is not be caught by this code, and can consequently be managed by code more specifically designed to handle it.
Noncompliant Code Example
In this noncompliant code example, a divide by zero exception was is handled initially. Instead of the specific exception type ArithmeticException
, a more generic type Exception
was is caught. This is dangerous since as any future exception declaration updates to the method signature (such as, addition of IOException
here) may no longer require the developer to provide a handler. Consequently, the recovery process may not be tailored to the specific exception type that gets is thrown. Additionally, unchecked exceptions under RuntimeException
are also unintentionally caught when whenever the top level Exception
class is caught.
...
Wiki Markup |
---|
*EXC32-J-EX1*: A secure application must also abide by [EXC01-J. Do not allow exceptions to transmit sensitive information]. In orderTo to follow this rule, an application might find it necessary to catch all exceptions at some 'top' -level to sanitize (or suppress) them. This is also summarized in the CWE entries, [CWE 7|http://cwe.mitre.org/data/definitions/7.html] and [CWE 388|http://cwe.mitre.org/data/definitions/388.html]. If exceptions need to be caught, it is better to catch {{Throwable}} instead of {{Exception}} \[[Roubtsov 03|AA. Java References#Roubtsov 03]\]. |
Risk Assessment
Catching RuntimeException
will trap traps several types of exceptions not intended to be caught. This prevents them from being handled properly.
...