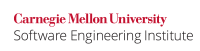
...
Explicitly check the range of each arithmetic operation and throw an ArithmeticException
on overflow; or else downcast the value to an integer. When performing operations on values of type int
or smaller, the arithmetic can be done using variables of type long
. For performing arithmetic operations on large numbers of type long
, the BigInteger
Class must be used.
...
_ _ -the integer data type is a 32-bit signed two's complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).
_ _ - the long
data type is a 64-bit signed two's complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use this data type when you need a range of values wider than those provided by int.
Since a variable of the long
type is guaranteed to hold the result of an int
additionaddition, subtraction or multiplication of values of type int
, the result can be assigned to a variable of type long
, and if the result is in the integer range, we can simply downcast to an a value of type int
.
Compliant Solution (Use
...
long
and Downcast)
This compliant solution uses the a variable of type long
type to store the result of the addition and proceeds to range check the value. If the value cannot be represented safely as an a value of type int
, it throws an ArithmeticException
. Otherwise, it downcasts the result to an a value of type int
.
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { long temp = (long)a + (long)b; if(temp >Integer.MAX_VALUE || temp < Integer.MIN_VALUE) throw new ArithmeticException(); else // Value within range can perform the addition return (int)temp; } |
Compliant Solution (Bounds Checking)
This compliant solution ensures that a
and b
variables are always positive and uses range checking to ensure that the result can be safely represented as an int
will not overflow.
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { int temp; if(a>0 && (b>0 && (a > >IntegerInteger.MAX_VALUE - b)) || a<0 && b<0 && (a < Integer.MIN_VALUE -b) ) throw new ArithmeticException(); else temp = a + b; //Value within range can perform the addition //Do stuff return temp; } |
...
Code Block | ||
---|---|---|
| ||
public boolean overflow(intlong a, intlong b) { BigInteger ba = new java.math.BigInteger(String.valueOf(a)); BigInteger bb = new java.math.BigInteger(String.valueOf(b)); BigInteger br = ba.add(bb); return if(br.compareTo(BigInteger.valueOf(IntegerLong.MAX_VALUE)) == 1 || || brbr.compareTo(BigInteger.valueOf(IntegerLong.MIN_VALUE))== -1) return true;// Overflow // Else no overflow or underflow return false; } public int do_operation(intlong a, intlong b) throws ArithmeticException { if(overflow(a,b)) throw new ArithmeticException(); else // Within range; safely perform the addition } |
With use of the BigInteger
class, integer overflows are definitely eliminated. However, due to increased performance costs, it should be used only on large operations or when limited memory is not a concernwhen other methods are not appropriate.
Subtraction
Care must be taken while performing the subtraction operation as well since overflows (or underflows) are still possible.
...
In this noncompliant code example, the subtraction operation may underflow overflow negatively when a
is a negative integer and b
is a large positive integer such that their sum is not representable as an a value of type int
. It can even also overflow when a
is positive whereas and b
is negative and their sum is not representable as an a value of type int
.
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) { int temp = a - b; // Could result in overflow // Perform other processing return temp; } |
...
Code Block | ||
---|---|---|
| ||
public int do_operation(int a,int b) { long temp = (long)a - (long)b; if(temp < Integer.MIN_VALUE || temp > Integer.MAX_VALUE) VALUE) throw new ArithmeticException(); else result = (int) temp; return temp; } |
Compliant Solution (Bounds Checking)
This compliant solution uses range checking to ensure that the result will not overflow.
Code Block | ||
---|---|---|
| ||
public int do_operation(int a, int b) throws ArithmeticException { int temp; if( (b>0 && (a < Integer.MIN_VALUE + b)) || (a > Integer.MAX_VALUE + b) ) throw new ArithmeticException(); else result temp = (int) temp; returna - b; //Value within range can perform the addition //Do stuff return temp; } |
Compliant Code Example (Use BigInteger Class)
A The BigInteger
class can be used as a test-wrapper as shown in this compliant solution.
Code Block | ||
---|---|---|
| ||
public boolean underflow(intlong a, intlong b) { BigInteger ba = new BigInteger(String.valueOf(a)); BigInteger bb = new BigInteger(String.valueOf(b)); BigInteger br = ba.subtract(bb); return if(br.compareTo(BigInteger.valueOf(IntegerLong.MAX_VALUE)) == 1 || || br.compareTo(BigInteger.valueOf(IntegerLong.MIN_VALUE)) == -1) return true;// Underflow // No underflow or overflow return false; } public int do_operation(intlong a, intlong b) throws ArithmeticException { if(underflow(a,b)) throw new ArithmeticException(); else // Within range; safely perform the subtraction } |
...
Since the size of the type long
(64 bits) is twice the size of the type int
(32 bits), the multiplication should be performed in terms using a variable of type long
. If the product is in the valid integer rangethe range of the int
type, it can be safely downcast to an a value of type int
.
Code Block | ||
---|---|---|
| ||
int a,b,result; long temp = (long) a\ * (long)b; if(temp > Integer.MAX_VALUE || temp < Integer.MIN_VALUE) throw new ArithmeticException(); // Overflow else result = (int) temp; // Value within range, safe to downcast |
...
A related pitfall is the use of the Math.abs()
method that takes an a parameter of type int
parameter and returns its absolute value. Because of the asymmetry between the representation of negative and positive integer values (there is an extra minimum negative value -128), there is no equivalent positive value (+128) for Integer.MIN_VALUE. As a result, Math.abs(Integer.MIN_VALUE)
always returns a non positive Integer.MIN_VALUE
.
...
- In C/C++ if the value being left shifted is negative or the right-hand operator of the shift operation is negative or greater than or equal to the width of the promoted left operand, there is undefined behavior. This does not extend to Java as integer types are masked by the last 5 lower . If the value to be shifted is of type
int
, only the five lowest-order bits of the right-hand operand (or are used as the shift distance. That is, the shift distance is the value of the right-hand operand masked by 31 (0x1F). This results in a value modulo 31, inclusive.
Wiki Markup When the value to be shifted (left-operand) is aof type {{long}}, only the last 6 bits of the right-hand operand are used to perform the shift. The shift distance is the value of the right-hand operand masked by 63 (0x3D) \[[JLS 03|AA. Java References#JLS 03]\], i.e., it is always between 0 and 63. (If the shift value is greater than 64, then the shift is {{value % 64}}.)
Refer to INT36-J. Use shift operators correctly for further details about the behavior of the shift operators.
...
Wiki Markup |
---|
In this example, the programmer wishes to shift the integervalue {{i}} of type {{int}} until, after 32 iterations, the value becomes 0. Unfortunately, this loop never terminates as an attempt to shift an a value of type {{int}} value by 32 bits results in the original {{int}} value rather than the value 0. \[[Bloch 05|AA. Java References#Bloch 05]\] |
...
Compliant Solution
This compliant solution repeatedly shifts initially sets the value val
to -1 with a different shift distance by saving the result of the previous shift and continuing to shift it bit by bit and repeatedly shifts the value by one place on each successive iteration.
...