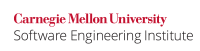
...
Code Block | ||
---|---|---|
| ||
import java.io.IOException; import java.io.BufferedReader; import java.io.FileReader; public class Login { static void checkPassword(String password_file) throws IOException { StringBuffer fileData = new StringBuffer(1000); BufferedReader reader = new BufferedReader(new FileReader(password_file)); try { int n; char[] passwd = new char[1024]; while ((n = reader.read(passwd)) >= 0) { String readData = String.valueOf(passwd, 0, n); fileData.append(readData); passwd = new char[1024]; } String realPassword = "javac<at:var at:name="f3b" />b3"; System.out.println(fileData.toString()); if (fileData.toString().equals(realPassword)) { System.out.println("Login successful"); } else { System.out.println("Login failed"); } } finally { try { //enclose in try-catch block reader.close(); //other clean-up code }catch (IOException ie) {ie.getMessage();} } } public static void main(String[] args) throws IOException { String path = "c:\\password.txt"; checkPassword(path); } } |
Compliant Solution 2
If the need to close a stream without throwing an exception occurs often, then an alternative solution to wrapping every call of close()
in its own try-catch
block is to write a method, as shown in this compliant solution.
Code Block | ||
---|---|---|
| ||
import java.io.IOException;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.Closeable;
public class Login {
static void checkPassword(String password_file) throws IOException {
StringBuffer fileData = new StringBuffer(1000);
BufferedReader reader = new BufferedReader(new FileReader(password_file));
try {
int n;
char[] passwd = new char[1024];
while ((n = reader.read(passwd)) >= 0) {
String readData = String.valueOf(passwd, 0, n);
fileData.append(readData);
passwd = new char[1024];
}
String realPassword = "javac<at:var at:name="f3b" />b3";
System.out.println(fileData.toString());
if (fileData.toString().equals(realPassword)) {
System.out.println("Login successful");
}
else {
System.out.println("Login failed");
}
} finally {
closeIgnoringException(reader);
//other clean-up code
}
}
private static void closeIgnoringException(Closeable s) {
if (s != null) {
try {
s.close();
} catch (IOException ie) {
// Ignore exception if close fails
}
}
}
public static void main(String[] args) throws IOException {
String path = "c:\\password.txt";
checkPassword(path);
}
}
|
Risk Assessment
Failing to handle an exception in a finally
block can lead to unexpected results.
...