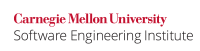
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // Down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream("input.dat")); System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.outerr.println("ERROR"); // fowardForward to handler } } } |
Implementation Details (POSIX)
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is to advance to the next line // The noncompliant code example deceptively // appeared to work without it (in some cases) return (char)input; } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.outerr.println("ERROR"); // Forward to handler } } } |
Implementation Details (POSIX)
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="691ef3594d27fc5d-cb737ac4-4d544070-a12d9e35-fad4d6f65f303c3b2b13459d"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | [method read | http://java.sun.com/javase/6/docs/api/java/io/InputStream.html#read()] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="9bfac9349d609b7a-0cbba482-494d4ce5-8e3cb7fe-c392329b3aad65370ad08a7b"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | [class BufferedInputStream | http://java.sun.com/javase/6/docs/api/java/io/BufferedInputStream.html] | ]]></ac:plain-text-body></ac:structured-macro> |
...