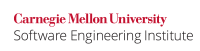
...
This noncompliant code example uses a floating-point variable as a loop counter. The decimal number 0.1 cannot be precisely represented as a float
or even as a double
.
Code Block | ||
---|---|---|
| ||
for (float x = 0.1f; x <= 1.0f; x += 0.1f) {
System.out.println(x);
}
|
...
This compliant solution uses an integer loop counter from which the desired floating-point value is derived.
Code Block | ||
---|---|---|
| ||
for (int count = 1; count <= 10; count += 1) {
float x = count/10.0f;
System.out.println(x);
}
|
...
This noncompliant code example uses a floating-point loop counter that is incremented by an amount that is typically too small to change its value given the precision.
Code Block | ||
---|---|---|
| ||
for (float x = 100000001.0f; x <= 100000010.0f; x += 1.0f) {
/* ... */
}
|
...
This compliant solution uses an integer loop counter from which the floating-point value is derived. Additionally, it uses a double
to ensure that the available precision suffices to represent the desired values. The solution also runs in FP-strict mode to guarantee portability of its results. See NUM06-J. Use the strictfp modifier for floating-point calculation consistency across platforms for more information.
Code Block | ||
---|---|---|
| ||
for (int count = 1; count <= 10; count += 1) {
double x = 100000000.0 + count;
/* ... */
}
|
...
Puzzle 34. Down for the count | |
[JLS 2005] | |
[Seacord 2015] | NUM09-J. Do not use floating-point variables as loop counters LiveLesson |