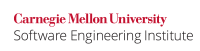
...
This noncompliant code example attempts to count the number of indices in arrays list1
and list2
that have equivalent values. Recall that class Integer
is required to memoize only those integer values in the range −128 to 127; it might return a nonunique object for any value outside that range. Consequently, when comparing autoboxed integer values outside that range, the ==
operator might return false and the example could deceptively output 0.
...
Code Block | ||
---|---|---|
| ||
public class Wrapper { public static void main(String[] args) { // Create an array list of integers ArrayList<Integer> list1 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list1.add(i + 1000); } // Create another array list of integers, where each element // has the same value as the first one ArrayList<Integer> list2 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list2.add(i + 1000); } // Count matching values int counter = 0; for (int i = 0; i < 10; i++) { if (list1.get(i).equals(list2.get(i))) { // usesUses 'equals()' counter++; } } // Print the counter: 10 in this example System.out.println(counter); } } |
...