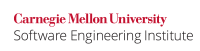
...
This noncompliant code example attempts to trim leading letters from the string
. It fails to accomplish this task because Character.isLetter()
lacks support for supplementary and combining characters [Hornig 2007]characters. Because it only examines one character at a time, this method also can corrupt combining characters.
Code Block | ||
---|---|---|
| ||
// Fails for supplementary or combining characters public static String trim(String string) { char ch; int i; for (i = 0; i < string.length(); i += 1) { ch = string.charAt(i); if (!Character.isLetter(ch)) { break; } } return string.substring(i); } |
...
This noncompliant code example attempts to correct corrects the problem with supplementary characters by using the String.codePointAt()
method, which accepts an int
argument. This works for supplementary characters but fails for combining characters [Hornig 2007]However, it still fails to handle combining characters because it only examines one character at a time.
Code Block | ||
---|---|---|
| ||
// Fails for combining characters public static String trim(String string) { int ch; int i; for (i = 0; i < string.length(); i += Character.charCount(ch)) { ch = string.codePointAt(i); if (!Character.isLetter(ch)) { break; } } return string.substring(i); } |
...
This compliant solution works both for supplementary and for combining characters [Hornig 2007Tutorials 2008]. According to the Java API [API 2006] class java.text.BreakIterator
documentation:
...
[API 2006] | Classes |
Problem Areas: CharactersCharacter Boundaries |