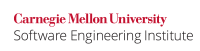
...
This noncompliant code example exposes instances of the someObject
SomeObject
class to untrusted code.
Code Block | ||
---|---|---|
| ||
public class SomeObject { public synchronized void changeValue() { // Locks on the object's monitor // ... } } // Untrusted code SomeObject someObject = new SomeObject(); synchronized (someObject) { while (true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject } } |
The untrusted code attempts to acquire a lock on the objectâs monitor and, upon succeeding, introduces an indefinite delay that prevents the synchronized
changeValue()
method from acquiring the same lock. Note that in the untrusted code, the attacker intentionally violates guideline LCK09-J. Do not perform operations that may block while holding a lock.
Noncompliant Code Example (
...
Public
Non-Final Lock Object)
This noncompliant code example locks on a public non-final object in an attempt to use a lock other than {{SomeObject}}âs intrinsic lock.
...
This noncompliant code example exposes the class object of someObject
SomeObject
to untrusted code.
Code Block | ||
---|---|---|
| ||
public class SomeObject { //changeValue locks on the class object's monitor public static synchronized void ChangeValuechangeValue() { // ... } } // Untrusted code synchronized (SomeObject.class) { while (true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject } } |
...
Code Block | ||
---|---|---|
| ||
public class SomeObject { private static final Object lock = new Object(); // private final lock object public static void ChangeValuechangeValue() { synchronized (lock) { // Locks on the private Object // ... } } } |
In this compliant solution, ChangeValuechangeValue()
obtains a lock on a private static Object
that is inaccessible to the caller.
...