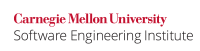
...
It
...
is
...
inappropriate
...
to
...
lock
...
on
...
an
...
object
...
of
...
a
...
class
...
that
...
implements
...
one
...
or
...
both
...
of
...
the
...
following
...
interfaces
...
of
...
the
...
java.util.concurrent.locks
...
package:
...
Lock
...
and
...
Condition
...
.
...
Using
...
the
...
intrinsic
...
locks
...
of
...
these
...
classes
...
is
...
a
...
questionable
...
practice
...
even
...
though
...
the
...
code
...
may
...
appear
...
to
...
function
...
correctly.
...
This
...
problem
...
is
...
commonly
...
discovered
...
when
...
code
...
is
...
refactored
...
from
...
intrinsic
...
locking
...
to
...
the
...
java.util.concurrent
...
dynamic
...
-locking
...
utilities.
...
Noncompliant
...
Code
...
Example
...
(
...
ReentrantLock
...
Lock
...
Object)
...
The
...
doSomething()
...
method
...
in
...
this
...
noncompliant
...
code
...
example
...
synchronizes
...
on
...
the
...
intrinsic
...
lock
...
of
...
an
...
instance
...
of
...
ReentrantLock
...
instead
...
of
...
the
...
reentrant
...
mutual
...
exclusion
...
Lock
...
encapsulated
...
by
...
ReentrantLock
...
.
Code Block | ||||
---|---|---|---|---|
| =
| |||
} private final Lock lock = new ReentrantLock(); public void doSomething() { synchronized(lock) { // ... } } {code} h2. Compliant Solution ({{ |
Compliant Solution (lock()
...
and
...
unlock()
...
)
...
Instead
...
of
...
using
...
the
...
intrinsic
...
locks
...
of
...
objects
...
that
...
implement
...
the
...
Lock
...
interface,
...
such
...
as
...
ReentrantLock
...
,
...
use
...
the
...
lock()
...
and
...
unlock()
...
methods
...
provided
...
by
...
the
...
Lock
...
interface.
Code Block | ||||
---|---|---|---|---|
| =
| |||
} private final Lock lock = new ReentrantLock(); public void doSomething() { lock.lock(); try { // ... } finally { lock.unlock(); } } {code} |
If
...
there
...
is
...
no
...
requirement
...
for
...
using
...
the
...
advanced
...
functionality
...
of
...
the
...
java.util.concurrent
...
package's
...
dynamic
...
-locking
...
utilities,
...
it
...
is
...
better
...
to
...
use
...
the
...
Executor
...
framework
...
or
...
other
...
concurrency
...
primitives
...
such
...
as
...
synchronization
...
and
...
atomic
...
classes.
...
Risk
...
Assessment
...
Synchronizing
...
on
...
the
...
intrinsic
...
lock
...
of
...
high-level
...
concurrency
...
utilities
...
can
...
cause
...
nondeterministic behavior
...
because
...
the
...
class
...
can
...
end
...
up
...
with
...
two
...
different
...
locking
...
policies.
...
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
LCK03-J | medium | probable | medium | P8 | L2 |
References
Wiki Markup |
---|
\[[API 2006|AA. Java References#API 06]\] \[[Findbugs |
...
2008|AA. Java References#Findbugs 08]\] \[[Pugh |
...
2008|AA. Java References#Pugh 08]\] "Synchronization" \[[Miller |
...
2009|AA. Java References#Miller 09]\] Locking \[[Tutorials |
...
2008|AA. Java References#Tutorials 08]\] [Wrapper Implementations|http://java.sun.com/docs/books/tutorial/collections/implementations/wrapper.html] |
...
...