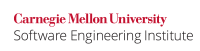
...
The monitoring application is built upon class Race
which maintains a list of racers. To be thread-safe, it locks each racer before it reads her statistics until after it reports the average.
Code Block | ||
---|---|---|
| ||
public class Race { static final static int MAX = 20; private static final Racer[] racers = new Racer[MAX]; public Race() { for (int i = 0; i < MAX; i++) { racers[i] = new Racer(/* initialize racer */); } } double getAverageCurrentSpeed() { return averageCurrentSpeedCalculator(0, 0.0); } double averageCurrentSpeedCalculator(int i, double currentSpeed) { // Acquires locks in increasing order if (i > MAX - 1) { return currentSpeed / MAX; } synchronized(racers[i]) { currentSpeed += racers[i].getCurrentSpeed(); return averageCurrentSpeedCalculator(++i, currentSpeed); } } double getAverageDistance() { return averageDistanceCalculator(MAX - 1, 0.0); } double averageDistanceCalculator(int i, double distance) { // Acquires locks in decreasing order if (i <= -1) { return distance / MAX; } synchronized(racers[i]) { distance += racers[i].getDistance(); return averageDistanceCalculator(--i, distance); } } } |
...