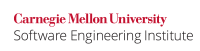
...
In this noncompliant example, security manager checks are used within the constructor but are not replicated throughout, specifically, within the readObject
and writeObject
methods that are used in the serialization-deserialization process. This allows an attacker to maliciously create instances of the class that bypass security manager checks when deserialization is performed.
Code Block | ||
---|---|---|
| ||
import java.io.Serializable; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public final class CreditCard implements java.io.Serializable { //private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; public CreditCard() { //initialize credit_card to default value credit_card = DEFAULT; } //allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // no change return; } else { validateInput(newCC); credit_card = newCC; } } // readObject correctly enforces checks during deserialization private void readObject(java.io.ObjectInputStream in) { in.defaultReadObject(); // if the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { validateInput(credit_card); } } // allows callers to retrieve internal state public String getValue() { return somePublicValue; } // writeObject correctly enforces checks during serialization private void writeObject(java.io.ObjectOutputStream out) { out.writeObject(credit_card); } } |
...