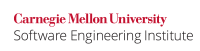
...
This compliant solution uses a private object lock to synchronize the method bodies of the increment()
and getCount
methods, to ensure atomicity. For more information on private object locks, see CON04-J. Use the private lock object idiom instead of method synchronizationthe Class object's intrinsic locking mechanism.
Code Block | ||
---|---|---|
| ||
public class KeyedCounter { private final Map<String,Integer> map = new HashMap<String,Integer>(); private final Object lock = new Object(); public void increment(String key) { synchronized (lock) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue() + 1; map.put(key, value); } } public Integer getCount(String key) { synchronized (lock) { return map.get(key); } } } |
...