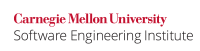
...
Code Block | ||
---|---|---|
| ||
class BankAccount { private static int balanceAmount; // Total amount in bank account private static final Object lock; private BankAccount(int balance) { this.balanceAmount = balance; this.lock = new Object(); } // Deposits the amount from this object instance to BankAccount instance argument ba private void depositAllAmount(BankAccount ba) { synchronized (lock) { ba.balanceAmount += this.balanceAmount; this.balanceAmount = 0; // withdraw all amount from this instance ba.displayAllAmount(); // Display the new balanceAmount in ba (may cause deadlock) } } private void displayAllAmount() { synchronized (lock) { System.out.println(balanceAmount); } } public static void initiateTransfer(final BankAccount first, final BankAccount second) { Thread t = new Thread(new Runnable() { public void run() { first.depositAllAmount(second); } }); t.start(); } } |
...