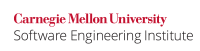
...
Code Block | ||
---|---|---|
| ||
public class TestWrapper2 { Â public public static void main(String[] args) { Â Â Â Â Integer     Integer i1 = 100; Â Â Â Â      Integer i2 = 100; Â Â Â Â      Integer i3 = 1000; Â Â Â Â      Integer i4 = 1000; Â Â Â Â      System.out.println(i1==i2); Â Â Â Â      System.out.println(i1!=i2); Â Â Â Â      System.out.println(i3==i4); Â Â Â Â      System.out.println(i3!=i4); Â Â Â Â Â       } } |
Output of this code
Here the cache in the Integer class can only make the number from -127 to 128 refer to the same object, which clearly explains the result of above code. In case of making such mistakes, when we need to do some comparisons of these wrapper class, we should use equal instead "==" (see EXP03-J for details):
...
Code Block | ||
---|---|---|
| ||
public class TestWrapper2 { Â public public static void main(String[] args) { Â Â Â Â Integer     Integer i1 = 100; Â Â Â Â      Integer i2 = 100; Â Â Â Â      Integer i3 = 1000; Â Â Â Â      Integer i4 = 1000; Â Â Â Â      System.out.println(i1.equals(i2)); Â Â Â Â      System.out.println(i3.equals(i4));Â Â Â Â Â ;      } }Â   |
Noncompliant Code Example
...
Code Block | ||
---|---|---|
| ||
import java.util.ArrayList; public class TestWrapper1 {  public public static void main(String[] args) {     //create an array list of integers, which each element     //is more than 127          ArrayList<Integer> list1 = new ArrayList<Integer>();          for(int i=0;i<10;i++)      list1      list1.add(i+1000);     //create another array list of integers, which each element     //is the same with the first one          ArrayList<Integer> list2 = new ArrayList<Integer>();          for(int i=0;i<10;i++)      list2      list2.add(i+1000);                ;                   int counter = 0;          for(int i=0;i<10;i++)      if      if(list1.get(i) == list2.get(i)) counter++;          //output the total equal number          System.out.println(counter);   } }   |
In JDK 1.6.0_10, the output of this code is 0. In this code, we want to count the same numbers of array list1 and array list2. Undoubtedly, the result is not the same as our expectation. Owning to the fact that the Integer can only caches from -127 to 128, so when int number beyond this range, it will be autoboxed into different objects, then the "==" will return false. But if we can set more caches inside Integer (cache all the integer value (-32K-32K), which means that all the int value could be autoboxed to the same Integer object) then the result may be different!
...
Code Block | ||
---|---|---|
| ||
public class TestWrapper1 {  public public static void main(String[] args) {     //create an array list of integers, which each element     //is more than 127          ArrayList<Integer> list1 = new ArrayList<Integer>();          for(int i=0;i<10;i++)      list1      list1.add(i+1000);     //create another array list of integers, which each element     //is the same with the first one          ArrayList<Integer> list2 = new ArrayList<Integer>();          for(int i=0;i<10;i++)      list2      list2.add(i+1000);                                   int counter = 0;          for(int i=0;i<10;i++)      if      if(list1.get(i).equals(list2.get(i))) counter++;          //output the total equal number          System.out.println(counter);   } } |
In JDK 1.6.0_10, the output of this code is 10.(the reason is the same as the above code example.)
...
Chapter 5, Core Java⢠2 Volume I - Fundamentals, Seventh Edition by Cay S. Horstmann, Gary Cornell
Publisher:Prentice Hall PTR;Pub Date:August 17, 2004.
Section 5.1.7, The Java⢠Language Specification,Third Edition by James Gosling, Bill Joy, Guy Steele, Gilad Bracha
Publisher:ADDISON-WESLEY;Pub Date:May 2005.
...
EXP04-J. Be wary of invisible implicit casts 02. Expressions (EXP) 03. Scope (SCP)