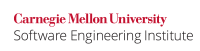
...
The best way to handle exceptions at a global level is to use an exception handler. The handler can perform diagnostic actions, clean-up and shutdown the Java Virtual Machine (JVM) or simply log the details of the failure. This guideline may be violated if the code for all runnable and callable tasks has been audited to ensure that no exceptional conditions are possible. Nonetheless, it is usually a good practice to install a task specific or global exception handler to initiate recovery, or log the exceptional condition.
Noncompliant Code Example (Abnormal task termination)
This noncompliant code example consists of class PoolService
that encapsulates a thread pool and a runnable class , Task
. The run()
method of the task can throw runtime exceptions such as NullPointerException
.
...
This compliant solution uses a Future
object to catch any exception thrown by the task. It uses the ExecutorService.submit()
method to submit the task so that a Future
object can be obtained.
Code Block | ||
---|---|---|
| ||
final class PoolService { private final ExecutorService pool = Executors.newFixedThreadPool(10); public void doSomething() { Future<?> future = pool.submit(new Task()); // ... try { future.get(); } catch (InterruptedException e) { Thread.currentThread().interrupt(); // Reset interrupted status } catch (ExecutionException e) { Throwable exception = e.getCause(); // Forward to exception reporter } } } |
Any exception that precludes doSomething()
from obtaining the Future
value can be handled as required.
Compliant Solution (ThreadPoolExecutor
hooks)
...