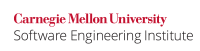
...
Code Block | ||
---|---|---|
| ||
class ReturnRef { // ... private Hashtable<Integer,String> getValues(){ return (Hashtable<Integer, String>)ht.clone(); // shallow copy } public static void main(String[] args) { ReturnRef rr = new ReturnRef(); Hashtable<Integer,String> ht1 = rr.getValues(); // prints non sensitive data ht1.remove(1); // untrusted caller can remove entries only from the copy Hashtable<Integer,String> ht2 = rr.getValues(); // prints non sensitive data } } |
If the hash table contained references to mutable data such as a series of Date
objects, every one of those objects must be copied by using a copy constructor or method. For further details, refer to FIO00-J. Defensively copy mutable inputs and mutable internal components and OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely. Note that the keys of a hash table need not be deep copied; shallow copying of the references suffices because a hash table's contract dictates that it cannot hold duplicate keys.
...
Code Block | ||
---|---|---|
| ||
class MutableClass { private Date d; public MutableClass() { d = new Date(); } protectedpublic Date getDate() { return d; } } |
...
If the class has a public
setter method it must follow related advice as given in FIO00-J. Defensively copy mutable inputs and mutable internal components. Note that a setter method may perform input validation and sanitization before setting the internal fields. On the other hand, returning references to internal objects may not require the caller to incorporate any of these defensive measures.
Noncompliant Code Example (mutable member array)
This noncompliant code example consists of an array of Date
objects.
Code Block | ||
---|---|---|
| ||
class MutableClass {
private Date[] date;
public MutableClass() {
for(int i = 0; i < 20; i++)
date[i] = new Date();
}
public Date[] getDate() {
return date; // or return date.clone()
}
}
|
It does not defensively copy the array before returning it. A shallow copy of the array may also be inappropriate in this case because Date
is mutable.
Compliant Solution (deep copying)
This compliant solution creates a deep copy of the date
array and returns the copy instead of the internal date
array.
Code Block | ||
---|---|---|
| ||
class MutableClass {
private Date[] date;
public MutableClass() {
for(int i = 0; i < 20; i++) {
date[i] = new Date();
}
}
public Date[] getDate() {
Date[] dates = new Date[20];
for(int i = 0; i < 20; i++) {
dates[i] = (Date) date[i].clone();
}
return dates;
}
}
|
Exceptions
Wiki Markup |
---|
*EX1:* According to Sun's Secure Coding Guidelines document \[[SCG 07|AA. Java References#SCG 07]\]: |
...