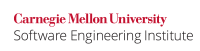
...
This noncompliant code example exposes the object someObject
to untrusted code. The untrusted code attempts to acquire a lock on the object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON06-J. Do not defer a thread that is holding a lock.
Code Block | ||
---|---|---|
| ||
public class SomeObject { public synchronized void changeValue() { // Locks on the object's monitor // ... } } // Untrusted code synchronized (someObject) { while (true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject } } |
The untrusted code attempts to acquire a lock on the object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON06-J. Do not defer a thread that is holding a lock.
Compliant Solution
Thread-safe classes that use intrinsic synchronization of the object may be protected by using the private lock object idiom and adapting them to use block synchronization. In this compliant solution, if the method changeValue()
is called, the lock is obtained on a private
Object
that is inaccessible from the caller.
...
This noncompliant code example exposes the class object of someObject
to untrusted code. The untrusted code attempts to acquire a lock on the class object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON06-J. Do not defer a thread that is holding a lock.
Code Block | ||
---|---|---|
| ||
public class SomeObject { public static synchronized void ChangeValue() { // Locks on the class object's monitor // ... } } // Untrusted code synchronized (someObject.getClass()) { while (true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject } } |
The untrusted code attempts to acquire a lock on the class object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON06-J. Do not defer a thread that is holding a lock.
Compliant Solution
Thread-safe classes that use intrinsic synchronization of the class object may be protected by using a static private lock object idiom and adapting them to use block synchronization. In this compliant solution, if the method ChangeValue()
is called, the lock is obtained on a static
private
Object
that is inaccessible from the caller. andblock synchronization.
Code Block | ||
---|---|---|
| ||
public class SomeObject { private static final Object lock = new Object(); // private lock object public static void ChangeValue() { synchronized (lock) { // Locks on the private Object // ... } } } |
In this compliant solution, if the method ChangeValue()
is called, the lock is obtained on a static
private
Object
that is inaccessible from the caller.
Using a private lock may only be achieved with block synchronization, as static method synchronization always uses the intrinsic lock of the object's class. However, block synchronization is also preferred over method synchronization, because it is easy to move operations out of the synchronized block when they might take a long time and they are not truly a critical section.
...