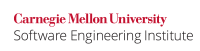
...
Code Block | ||
---|---|---|
| ||
final public class Point implements Cloneable { private int x; private int y; Point(int x, int y){ this.x = x; this.y = y; } void set_xy(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: "+ this.x); System.out.println("the value y is: "+ this.y); } public Point clone() throws CloneNotSupportedException{ Point cloned = (Point) super.clone(); cloned.x = this.x; cloned.y = this.y;// No need to clone x and y as they are primitives return cloned; } } public class PointCaller { public static void main(String[] args) throws CloneNotSupportedException { final Point point = new Point(1, 2); point.print_xy(); // Get the copy of original object Point pointCopy = point.clone(); // Change the value of x,y of the copy. pointCopy.set_xy(5, 6); // Original value remains unchanged point.print_xy(); } } |
...