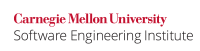
Note | ||
---|---|---|
| ||
This guideline has been deprecated. It has been merged with: 06/15/2015 -- Version 1.0 |
Similarly, a final
method parameter obtains an immutable copy of the object reference. Again, this has no effect on the mutability of the referenced data.
Noncompliant Code Example (Mutable Class, final
Reference)
In this noncompliant code example, the programmer has declared the reference to the point
instance to be final
under the incorrect assumption that doing so prevents modification of the values of the instance fields x
and y
. The values of the instance fields can be changed after their initialization because the final
clause applies only to the reference to the point
instance and not to the referenced object.
Code Block | ||
---|---|---|
| ||
class Point {
private int x;
private int y;
Point(int x, int y) {
this.x = x;
this.y = y;
}
void set_xy(int x, int y) {
this.x = x;
this.y = y;
}
void print_xy() {
System |
When you declare a variable final, you do not want anyone to change it. If the type of variable is primitive types, you can undoubtedly make it. Unfortunately, if the variable is a reference to an object, the "final" stuff you think may be not final!
Non-compliant Code Example
Code Block |
---|
class Test{ Â Â Test(int a, int b){ Â Â this.a = a; Â Â this.b = b; Â } Â void set_ab(int a, int b){ Â Â this.a = a; Â Â this.b = b; Â } Â void print_ab(){ Â Â System.out.println("the value ax is: " + this.ax); Â Â System System.out.println("the value by is: " + this.by); Â } Â private int a; Â private int b; }} } public class TestFinal1PointCaller { Â Â public public static void main(String[] args) { Â Â Â Â Â Â Â final TestPoint mytestpoint = new TestPoint(1, 2); Â Â Â Â Â Â Â mytestpoint.print_abxy(); Â Â Â Â Â Â Â //now we changeChange the value of ax,b. Â Â Â Â Â Â Â mytest y point.set_abxy(5, 6); Â Â Â Â Â Â Â mytestpoint.print_abxy(); Â Â Â Â Â Â Â Â Â Â } } |
...
Compliant Solution (final
Fields)
When the values of the x
and y
instance variables must remain immutable after their initialization, they should be declared final
. However, this invalidates a set_xy()
method because it can no longer change the values of x
and y
:
Code Block | ||
---|---|---|
| ||
class Point {
private final int x;
private final int y;
Point(int x, int y) {
this.x = x;
this.y = y;
}
void print_xy() {
System.out.println("the value x is: " + this.x);
System.out.println("the value y is: " + this.y);
}
// set_xy(int x, int y) no longer possible
}
|
With this modification, the values of the instance variables become immutable and consequently match the programmer's intended usage model.
Compliant Solution (Provide Copy Functionality)
If the class must remain mutable, another compliant solution is to provide copy functionality. This compliant solution provides a clone()
method in the class Point
, avoiding the elimination of the setter method:
Code Block | ||
---|---|---|
| ||
final public class Point implements Cloneable {
private int x;
private int y;
Point(int x, int y) {
this.x = x;
this.y = y;
}
void set_xy(int x, int y) {
this.x = x;
this.y = y;
}
void print_xy() {
System |
Non-Compliant Solution
If you do not want to change a and b after they are initialized, the simplest approach is to declare a and b final:
Code Block |
---|
 void set_ab(int a, int b){ //But now compiler complains about set_ab method!
  this.a = a;
  this.b = b;
 }
 private final int a;
 private final int b;
|
But now you can not have setter methods of a and b.
Compliant Solution
An alternative approach is to provide the clone method in the class. When you want do something about the object, you can use clone method to get a copy of original object. Now, you can do everything to this new object, and the original one will be never changed.
Code Block |
---|
class NewFinal implements Cloneable {  NewFinal(int a, int b){   this.a = a;   this.b = b;  }  void print_ab(){   System.out.println("the value ax is: "+ this.ax);   System System.out.println("the value by is: "+ this.by);  }  void set_ab(int a, int b){   this.a = a;   this.b = b;  }  public NewFinal } public Point clone() throws CloneNotSupportedException{   NewFinal Point cloned = (NewFinalPoint) super.clone();   return cloned;  }  private int a;  private int b; } // No need to clone x and y as they are primitives return cloned; } } public class Test2PointCaller {   public public static void main(String[] args) throws CloneNotSupportedException {        final NewFinalPoint mytestpoint = new NewFinalPoint(1, 2);        mytest // Is not changed in main() point.print_abxy();        //get Get the copy of original object    try {  NewFinal mytest2Point pointCopy = mytestpoint.clone();        //now wepointCopy changenow theholds valuea ofunique a,breference ofto the copy.  // newly  mytest2.set_ab(5, 6);   cloned Point instance //but Change the originalvalue valueof willx,y notof bethe changedcopy.        mytestpointCopy.printset_abxy(5, 6);   } catch (CloneNotSupportedException e) {    // TODOOriginal Auto-generatedvalue catchremains block    e.printStackTraceunchanged point.print_xy();   }  } } |
Risk Assessment
Using final to declare the reference to an object is a potential security risk, because the contents of the object can still be changed.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC37-J | medium | likely | low | P18 | L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
The clone()
method returns a copy of the original object that reflects the state of the original object at the moment of cloning. This new object can be used without exposing the original object. Because the caller holds the only reference to the newly cloned instance, the instance fields cannot be changed without the caller's cooperation. This use of the clone()
method allows the class to remain securely mutable. (See OBJ04-J. Provide mutable classes with copy functionality to safely allow passing instances to untrusted code.)
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object.
Applicability
Incorrectly assuming that final
references cause the contents of the referenced object to remain mutable can result in an attacker modifying an object believed to be immutable.
Bibliography
Item 13, "Minimize the Accessibility of Classes and Members" | |
Chapter 6, "Interfaces and Inner Classes" | |
[JLS 2013] |
...
Chapter 6, Core Java⢠2 Volume I - Fundamentals, Seventh Edition