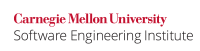
...
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200, 5); division(200, 0); // Divide by zero } catch (ArithmeticException | IOException ex) { ExceptionReporter.report(ex); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("Average: "+ average); } } |
Exceptions
ERR08-J-EX0: A catch
block may catch all exceptions to process them before rethrowing them (filtering sensitive information from exceptions before the call stack leaves a trust boundary, for example). Refer to ERR01-J. Do not allow exceptions to expose sensitive information and weaknesses CWE 7 and CWE 388 for more information. In such cases, a catch
block should catch Throwable
rather than Exception
or RuntimeException
.
...
Exception wrapping is a common technique to safely handle unknown exceptions. For another example, see ERR06-J. Do not throw undeclared checked exceptions.
ERR08-J-EX1: Task processing threads such as worker threads in a thread pool or the Swing event dispatch thread are permitted to catch RuntimeException
when they call untrusted code through an abstraction such as the Runnable
interface [Goetz 2006, p. 161].
ERR08-J-EX2: Systems that require substantial fault tolerance or graceful degradation are permitted to catch and log general exceptions such as Throwable
at appropriate levels of abstraction. For example:
...
Catching NullPointerException
may mask an underlying null dereference, degrade application performance, and result in code that is hard to understand and maintain. Likewise, catching RuntimeException
, Exception
, or Throwable
may unintentionally trap other exception types and prevent them from being handled properly.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR08-J | Medium | Likely | Medium | P12 | L1 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.STRUCT.EXCP.GEH | Generic Exception Handler (Java) | ||||||
Parasoft Jtest |
...
| CERT.ERR08.NCNPE | Do not catch 'NullPointerException' | |||||||
SonarQube |
| ||||||||
SpotBugs |
| DCN_NULLPOINTER_EXCEPTION | Implemented (since 4.5.0) |
...