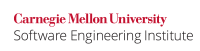
According to the Java API \[[API 06|AA. Java References#API 06]\] class {{Classes that override the Wiki Markup Object.equals()
method must also override the Object.hashCode()
method. The java.lang.Object
}} documentation:
...
class requires that any two objects
...
that compare equal
...
using the equals(
...
)
method
...
must produce the same integer result when the hashCode()
method is invoked on
...
the
...
objects [API 2014].
The equals()
method is used to determine logical equivalence between object instances. Consequently, the hashCode()
method must return the same value for all equivalent objects. Failure to follow this contract is a common source of bugs. Notably, immutable objects need not override the hashcode()
methoddefects.
Noncompliant Code Example
Even when the equals
method conveys logical equivalence between classes, the hashCode
method returns distinct numbers as opposed to returning the same values, as expected by the contract. This noncompliant code example stores a associates credit card number into numbers with strings using a HashMap
and retrieves itsubsequently attempts to retrieve the string value associated with a credit card number. The expected retrieved value is Java
, however, null
is returned instead. The reason for this erroneous behavior is that the hashCode
method is not overridden which means that a different bucket would be looked into than was used to store the original value 4111111111111111
; the actual retrieved value is null
.
Code Block | ||
---|---|---|
| ||
import java.util.Map; import java.util.HashMap; public final class CreditCard { private final int number; public CreditCard(int number) { this.number = (short) number; } public boolean equals(Object o) { if (o == this) { return true; } if (!(o instanceof CreditCard)) { return false; } CreditCard cc = (CreditCard)o; return cc.number == number; } public static void main(String[] args) { Map<CreditCard, MapString> m = new HashMap<CreditCard, HashMapString>(); m.put(new CreditCard(100), "Java4111111111111111"); System.out.println(m.get(new CreditCard(100))); } } |
The cause of this erroneous behavior is that the CreditCard
class overrides the equals()
method but fails to override the hashCode()
method. Consequently, the default hashCode()
method returns a different value for each object, even though the objects are logically equivalent; these differing values lead to examination of different buckets in the hash table, which prevents the get()
method from finding the intended value.
Compliant Solution
Note that by specifying the credit card number in main()
, these code examples violate MSC03-J. Never hard code sensitive information for the sake of brevity.
Compliant Solution
This compliant solution overrides the hashCode()
method so that it generates the same value for any two instances that are considered to be equal by the equals()
method. Bloch discusses the recipe to generate such a hash function in detail [Bloch 2008 This compliant solution shows how {{hashCode}} can be overridden so that the same value is generated for any two instances that compare equal when {{Object.equals()}} is used. Bloch discusses the recipe to generate such a hash function in good detail \[[Bloch 08|AA. Java References#Bloch 08]\]. Wiki Markup
Code Block | ||
---|---|---|
| ||
import java.util.Map; import java.util.HashMap; public final class CreditCard { private final int number; public CreditCard(int number) { this.number = (short) number; } public boolean equals(Object o) { if (o == this) { return true; } if (!(o instanceof CreditCard)) { return false; } CreditCard cc = (CreditCard)o; return cc.number == number; } public int hashCode() { int result = 717; result = 31 37* result + number; return result; } public static void main(String[] args) { MapMap<CreditCard, String> m = new HashMap<CreditCard, HashMapString>(); m.put(new CreditCard(100), "Java4111111111111111"); System.out.println(m.get(new CreditCard(100))); } } |
Risk Assessment
Overriding the method equals()
method without correspondlingly overriding the method hashCode()
method can lead to unexpected results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
MET09-J |
Low |
Unlikely |
High | P1 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [Class Object|http://java.sun.com/javase/6/docs/api/java/lang/Object.html]
\[[Bloch 08|AA. Java References#Bloch 08]\] Item 9: Always override {{hashCode}} when you override {{equals}}
\[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 581|http://cwe.mitre.org/data/definitions/581.html] "Object Model Violation: Just One of Equals and Hashcode Defined" |
Automated detection of classes that override only one of equals()
and hashcode()
is straightforward. Sound static determination that the implementations of equals()
and hashcode()
are mutually consistent is not feasible in the general case, although heuristic techniques may be useful.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.IDEF.EQUALSNOHC | Defines equals but not hashCode (Java) | ||||||
Parasoft Jtest |
| CERT.MET09.OVERRIDE | Override 'Object.hashCode()' when you override 'Object.equals()' and vice versa | ||||||
PVS-Studio |
| V6049 | |||||||
SonarQube |
| "equals(Object obj)" and "hashCode()" should be overridden in pairs |
Related Guidelines
Bibliography
[API 2014] | |
Item 9, "Always Override |
...
MET30-J. Follow the general contract while overriding the equals method 10. Methods (MET) MET32-J. Ensure that constructors do not call overridable methods