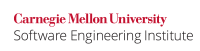
The write()
method that is , defined in the class java.io.OutputStream
, takes an integer argument intended to be between 0 and argument of type int
the value of which must be in the range 0 to 255. Because an int
is otherwise designed to store 4 byte numbers, failure to validate user input may lead to unexpected results.
Wiki Markup |
---|
The general contract for write is that one byte is written to the output stream. The byte to be written is the eight low-order bits of the argument b. The 24 high-order bits of b are ignored. \[[API 06|AA. Java References#API 06]\] |
Noncompliant Code Example
a value of type int
could be outside this range, failure to range check can result in the truncation of the higher-order bits of the argument.
The general contract for the write()
method says that it writes one byte to the output stream. The byte to be written constitutes the eight lower-order bits of the argument b
, passed to the write()
method; the 24 high-order bits of b
are ignored (see java.io.OutputStream.write()
[API 2014] for more information).
Noncompliant Code Example
This The noncompliant code example accepts a value from the user without validating it. If this Any value is greater than 255, it will result in a wrap aroundthat is not in the range of 0 to 255 is truncated. For instance, write(305)
will print '1' since the lower order bits of 305 are preserved while the top 24 order bits are lost (305 is 0x131 in Hex so the last Hex digit '1' is displayed303)
prints /
on ASCII-based systems because the lower-order 8 bits of 303 are used while the 24 high-order bits are ignored (303 % 256 = 47, which is the ASCII code for /
). That is, the result is the remainder modulo 256 of the absolute value of the input divided by 256.
Code Block | ||
---|---|---|
| ||
class ConsoleWrite { public static void main(String[] args) { // Any input value > 255 will result in unexpected output System.out.write(Integer.valueOf(args[0].toString())); System.out.flush(); } } |
Compliant Solution
...
(Range-Check Inputs)
This compliant solution prints the corresponding character only if the input integer is in the proper range. If the input is outside the representable range of an int
, the Integer.valueOf()
method throws a NumberFormatException
. If the input can be represented by an int
but is outside the range required by write()
, this code throws an ArithmeticException
Use alternative means to output integers such as the System.out.print*
methods.
Code Block | ||
---|---|---|
| ||
class ConsoleWriteFileWrite { public static void main(String[] args) { System.out.println(args[0]); } } |
Compliant Solution (2)
Alternatively, perform input validation. While this particular solution will still not display the integer
correctly, it will behave well when the corresponding read
method is utilized to convert the byte back to an integer
.
Code Block | ||
---|---|---|
| ||
class FileWrite { public static void main(String[] args) throws NumberFormatException, IOException { FileOutputStream out = new FileOutputStream("output.txt"); throws NumberFormatException, IOException { // Perform inputrange validation checking if(Integer.valueOf(args[0]) >= 0int && Integer.valueOf(args[0]) <= 255) { out.write(value = Integer.valueOf(args[0].toString())); if System.out.flush(); } else { //handle error (value < 0 || value > 255) { throw new ArithmeticException("Value is out of range"); } System.out.write(value); System.out.flush(); } } |
Compliant Solution (writeInt(
...
)
)
This compliant solution uses Similarly, if it is required to write a larger integer value, the writeInt()
method of the DataOutputStream
class can be used. Again, the output should not be used without appropriately escaping or encoding it., which can output the entire range of values representable as an int
:
Code Block | ||
---|---|---|
| ||
class FileWrite { public static void main(String[] args) throws NumberFormatException, IOException { FileOutputStream out = new FileOutputStream("output.txt"); throws NumberFormatException, IOException { DataOutputStream dos = new DataOutputStream(System.out); dos.writeInt(Integer.valueOf(args[0].toString())); dos.close(); System.out.closeflush(); } } |
Risk Assessment
Using the write()
method to output integers may outside the range 0 to 255 will result in unexpected valuestruncation.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
FIO09-J |
Low |
Unlikely |
Medium | P2 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [method write()|http://java.sun.com/javase/6/docs/api/java/io/OutputStream.html#write(int)]
\[[Harold 99|AA. Java References#Harold 99]\] |
Automated detection of all uses of the write()
method is straightforward. Sound determination of whether the truncating behavior is correct is not feasible in the general case. Heuristic checks could be useful.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.NULL.RET.UNCHECKED | Call Might Return Null (Java) | ||||||
Coverity | 7.5 | CHECKED_RETURN | Implemented | ||||||
Parasoft Jtest |
| CERT.FIO09.ARGWRITE | Do not rely on the write() method to output integers outside the range 0 to 255 |
Related Guidelines
Bibliography
[API 2014] | Class OutputStream |
...
INT30-J. Be careful while casting integers to narrower types 04. Integers (INT) INT32-J. Perform conversion from BigInteger to String and back properly