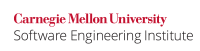
...
Code Block | ||
---|---|---|
| ||
public class SomeObject { // Locks on the object's monitor public synchronized void changeValue() { // ... } public static SomeObject lookup(String name) { // ... } } // Untrusted code String name = // ... SomeObject someObject = SomeObject.lookup(name); if (someObject == null) { // ... handle error } synchronized (someObject) { while (true) { // Indefinitely delaylock someObject Thread.sleep(Integer.MAX_VALUE); } } |
...
Code Block | ||
---|---|---|
| ||
public class SomeObject {
public final Object lock = new Object();
public void changeValue() {
synchronized (lock) {
// ...
}
}
}
// Untrusted code
SomeObject someObject = new SomeObject();
someObject.lock.wait();
|
Untrusted code that has the ability to create an instance of the class or has access to an already created instance can invoke the wait()
method on the publicly accessible lock
, causing the lock in the changeValue()
method to be released immediately. Furthermore, if the method were to invoke lock.wait()
from its body and not test a condition predicate, it would be vulnerable to malicious notifications. (See rule THI03-J. Always invoke wait() and await() methods inside a loop for more information.)
This noncompliant code example also violates rule OBJ01-J. Limit accessibility of fields.
...
LCK00-J-EX2: Package-private classes that are never exposed to untrusted code are exempt from this rule because their accessibility protects against untrusted callers. However, use of this exemption should be documented explicitly to ensure that trusted code within the same package neither reuses the lock object nor changes the lock object inadvertently.
...
Exposing the lock object to untrusted code can result in DoS.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
LCK00-J | low | probable | medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
The Checker Framework |
| Lock Checker | Concurrency and lock errors (see Chapter 6) | ||||||
CodeSonar |
| JAVA.CONCURRENCY.LOCK.ISTR | Synchronization on Interned String (Java) | ||||||
Parasoft Jtest |
| CERT.LCK00.SOPF | Do not synchronize on "public" fields since doing so may cause deadlocks | ||||||
SonarQube |
|
|
| S2445 |
Related Guidelines
Bibliography
Item 52. Document Thread Safety |
...