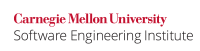
Java does not support the use of unsigned types, except for the 16 bit char
datatype. Sometimes, it is necessary to The only unsigned primitive integer type in Java is the 16-bit char
data type; all of the other primitive integer types are signed. To interoperate with native languages, such as C /or C++, that use unsigned types extensively. The standard practice to deal with unsigned types is to read their values into Java's larger signed
primitives, any unsigned values must be read and stored into a Java integer type that can fully represent the possible range of the unsigned data. For example, a signed long
is used to hold an unsigned integer
the Java long
type can be used to represent all possible unsigned 32-bit integer values obtained from native code.
Noncompliant Code Example
This noncompliant code example incorrectly uses a generic method for reading in integer data irrespective without considering the signedness of the signednesssource. It assumes that the value data read is always signed and thus treats the most significant bit (MSB) as the sign bit causing misinterpretations about . When the data read is unsigned, the actual sign and magnitude of the integervalues may be misinterpreted.
Code Block | ||
---|---|---|
| ||
public static int getInteger(DataInputStream is) throws IOException {
return is.readInt();
}
|
Compliant Solution
...
This compliant solution reads-in an unsigned integer value into an array of four bytes. The bytes are left shifted the appropriate amount and OR'ed together. However, when the bytes are shifted they are promoted to int. If the byte is negative, then all the left-most bits of the resulting int will be set, and these have to be masked off. Finally, the whole int expression may be negative when it is promoted to the long {{result}} and, again, the left-most bits have to be masked off. (cf. \[[Harold 97|AA. Java References#Harold 97]\] but the code there is wrong.)solution requires that the values read are 32-bit unsigned integers. It reads an unsigned integer value using the readInt()
method. The readInt()
method assumes signed values and returns a signed int
; the return value is converted to a long
with sign extension. The code uses an &
operation to mask off the upper 32 bits of the long
, producing a value in the range of a 32-bit unsigned integer, as intended. The mask size should be chosen to match the size of the unsigned integer values being read.
Code Block | ||
---|---|---|
| ||
public static long readIntgetInteger(InputStreamDataInputStream is) throws IOException { byte[] buffer = new byte[4]; int check = is.read(buffer); if (check != 4) throw new IOException("Unexpected End of Stream!"); long result = (buffer[0] << 24) | (0x00FFFFFF&(buffer[1] << 16)) | (0x0000FFFF&(buffer[2] << 8)) | (0x000000FF&buffer[3]); result &= 0xFFFFFFFFL; return result; } |
Risk Assessment
return is.readInt() & 0xFFFFFFFFL; // Mask with 32 one-bits
}
|
As a general principle, you should always be aware of the signedness of the data you are reading.
Risk Assessment
Treating unsigned data as though it were signed produces incorrect values and can lead to lost or misinterpreted dataTreating an unsigned type as signed can result in misinterpretations and can lead to erroneous calculations.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
NUM03-J |
Low |
Unlikely |
Medium |
P2 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class DataInputStream: method {{readInt}}
\[[Harold 97|AA. Java References#Harold 97]\] Chapter 2: Primitive Data Types, Cross Platform Issues, Unsigned Integers |
Automated detection is infeasible in the general case.
Bibliography
[API 2006] | Class |
Chapter 2, "Primitive Data Types, Cross-Platform Issues, Unsigned Integers" | |
Section 2.4.5, "Accessing Unsigned Data" | |
[Seacord 2015] |
...
INT00-J. Provide methods to read and write Little-Endian data 04. Integers (INT) 04. Integers (INT)