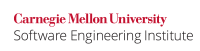
According to \[[JLS 05|AA. Java References#JLS 05]\]to The Java Language Specification, §12.5, "Creation of New Class Instances" [JLS 2015]: Wiki Markup
Unlike C++, the Java programming language does not specify altered rules for method dispatch during the creation of a new class instance. If methods are invoked that are overridden in subclasses in the object being initialized, then these overriding methods are used, even before the new object is completely initialized.
This means that a method may execute on uninitialized data and cause Invocation of an overridable method during object construction may result in the use of uninitialized data, leading to runtime exceptions or lead to unanticipated outcomes.
Noncompliant Code Example
Calling overridable methods from constructors can also leak the this
reference before object construction is complete, potentially exposing uninitialized or inconsistent data to other threads (see TSM01-J. Do not let the this reference escape during object construction for additional information). As a result, a class's constructor must invoke (directly or indirectly) only methods in that class that are static, final or private.
Noncompliant Code Example
This noncompliant code example results in the use of uninitialized data by the doLogic()
method:This noncompliant example invokes the doLogic
method from the constructor. The super class doLogic
method gets invoked on the first call, however, the overriding method is invoked on the second one. In the second call, the issue is that the constructor for SubClass
initiates the super class's constructor which undesirably ends up calling SubClass
's doLogic()
method. The value of color
is left as null
because the initialization of the class SubClass
has not yet concluded.
Code Block | ||
---|---|---|
| ||
class BaseClassSuperClass { public BaseClassSuperClass () { doLogic(); } public void doLogic() { System.out.println("This is super-classsuperclass!"); } } class SubClass extends BaseClassSuperClass { private String color = null; public SubClass() { super(); color = "Redred"; } public void doLogic() { System.out.println("This is sub-classsubclass! The color is :" + color); //color becomes null //other operations... } } public class Overridable { public static void main(String[] args) { BaseClassSuperClass bc = new BaseClassSuperClass(); //prints Prints "This is super-classsuperclass!" BaseClassSuperClass sc = new SubClass(); //prints Prints "This is sub-classsubclass! The color is :null" } } |
The doLogic()
method is invoked from the superclass's constructor. When the superclass is constructed directly, the doLogic()
method in the superclass is invoked and executes successfully. However, when the subclass initiates the superclass's construction, the subclass's doLogic()
method is invoked instead. In this case, the value of color
is still null
because the subclass's constructor has not yet concluded.
Compliant Solution
This compliant solution declares the doLogic()
method as final so that it is no longer overridable.cannot be overridden:
Code Block | ||
---|---|---|
| ||
class BaseClassSuperClass { public BaseClassSuperClass() { doLogic(); } public final void doLogic() { System.out.println("This is super-classsuperclass!"); } } |
In addition to constructors, do not call overridable methods from the clone
, readObject
and readObjectNoData
methods as it would allow attackers to obtain partially initialized instances of classes. An equally dangerous idea is to disobey this advice by calling an overridden method from a finalize
method. This can prolong the subclass' life and in fact render the finalization call useless (See the example in OBJ02-J. Avoid using finalizers). Additionally, if the subclass's finalizer has terminated key resources, invoking its methods from the superclass might lead one to observe the object in an inconsistent state and in the worst case result in the infamous NullPointerException
.
Risk Assessment
Allowing a constructor to call overridable methods may give can provide an attacker with access to the this
reference before an object is fully initialized, which , in turn, could lead to a vulnerability.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
MET05-J |
Medium |
Probable |
Medium | P8 | L2 |
Automated Detection
...
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] [Chapter 8, Classes|http://java.sun.com/docs/books/jls/third_edition/html/classes.html], 12.5 "Creation of New Class Instances"
\[[SCG 07|AA. Java References#SCG 07]\] Guideline 4-3 Prevent constructors from calling methods that can be overridden |
Automated detection of constructors that contain invocations of overridable methods is straightforward.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
PVS-Studio |
| V6052 | |||||||
SonarQube |
| S1699 | Constructors should only call non-overridable methods | ||||||
SpotBugs |
| MC_OVERRIDABLE_METHOD_CALL_IN_CONSTRUCTOR | Implemented (since 4.5.0) |
Related Guidelines
Inheritance [RIP] | |
Guideline 7-4 / OBJECT-4: Prevent constructors from calling methods that can be overridden |
Bibliography
[ESA 2005] | Rule 62, Do not call nonfinal methods from within a constructor |
[JLS 2015] | Chapter 8, "Classes" |
Rule 81, Do not call non-final methods from within a constructor |
...
MET31-J. Ensure that hashCode() is overridden when equals() is overridden 09. Methods (MET) 10. Exceptional Behavior (EXC)