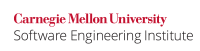
The C Standard, 6.7.3.2, paragraph 20 [ISO/IEC 9899:2024], says
As a special case, the last element of a structure with more than one named member may have an incomplete array type; this is called a flexible array member. In most situations, the flexible array member is ignored. In particular, the size of the structure is as if the flexible array member were omitted except that it may have more trailing padding than the omission would imply.
The following is an example of a structure that contains a flexible array member:
Code Block |
---|
struct flex_array_struct {
int num;
int data[];
};
|
This definition means that when computing the size of such a structure, only the first member, num
, is considered. Unless the appropriate size of the flexible array member has been explicitly added when allocating storage for an object of the struct
, the result of accessing the member data
of a variable of nonpointer type struct flex_array_struct
is undefined. DCL38-C. Use the correct syntax when declaring a flexible array member describes the correct way to declare a struct
with a flexible array member.
To avoid the potential for undefined behavior, structures that contain a flexible array member should always be allocated dynamically. Flexible array structures must
- Have dynamic storage duration (be allocated via
malloc()
or another dynamic allocation function) - Be dynamically copied using
memcpy()
or a similar function and not by assignment - When used as an argument to a function, be passed by pointer and not copied by value
Noncompliant Code Example (Storage Duration)
This noncompliant code example uses automatic storage for a structure containing a flexible array member:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stddef.h>
struct flex_array_struct {
size_t num;
int data[];
};
void func(void) {
struct flex_array_struct flex_struct;
size_t array_size = 4;
/* Initialize structure */
flex_struct.num = array_size;
for (size_t i = 0; i < array_size; ++i) {
flex_struct.data[i] = 0;
}
} |
Because the memory for flex_struct
is reserved on the stack, no space is reserved for the data
member. Accessing the data
member is undefined behavior.
Compliant Solution (Storage Duration)
This compliant solution dynamically allocates storage for flex_array_struct
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h>
struct flex_array_struct {
size_t |
Wiki Markup |
---|
Flexible array members are a special type of array where the last element of a structure with more than one named member has an incomplete array type; that is, the size of the array is not specified explicitly within the structure. A variety of different syntaxes have been used for declaring flexible array members. For C99-compliant implementations, use the syntax guaranteed valid by C99 \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\]. |
Non-Compliant Code Example
In this non-compliant code, an array of size 1 is declared, but when the structure itself is instantiated, the size computed for malloc()
is modified to account for the actual size of the dynamic array. This is the syntax used by ISO C89.
Code Block | ||
---|---|---|
| ||
struct flexArrayStruct { int num; int data[1]; }; /* ... */ /* Space is allocated void func(void) { struct flex_array_struct *flex_struct; size_t array_size = 4; /* Dynamically allocate memory for the struct */ struct flexArrayStruct *structPflex_struct = (struct flexArrayStructflex_array_struct *)malloc( sizeof(struct flexArrayStruct) flex_array_struct) + sizeof(int) * (ARRAY_SIZE - 1))array_size); if (structPflex_struct == NULL) { /* handleHandle mallocerror failure */ } structP->num = SOME_NUMBER; /* AccessInitialize data[] as if it had been allocated as data[ARRAY_SIZE] */ for (structure */ flex_struct->num = array_size; for (size_t i = 0; i < ARRAYarray_SIZEsize; i++i) { structP flex_struct->data[i] = i0; } |
Wiki Markup |
---|
The problem with this code is that the only member that is guaranteed to be valid, by strict C99 definition, is {{structP->data\[0\]}}. Consequently, for all {{i > 0}}, the results of the assignment are undefined. |
Implementation Details
The non-compliant example may be the only alternative for compilers that do not yet implement the C99 syntax. Microsoft Visual Studio 2005 does not implement the C99 syntax.
Compliant Solution
This compliant solution uses the flexible array member to achieve a dynamically sized structure.
} |
Noncompliant Code Example (Copying)
This noncompliant code example attempts to copy an instance of a structure containing a flexible array member (struct
) by assignment:flex_array_struct
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stddef.h>
struct flex_array_struct {
size_t num;
int data[];
};
void func(struct flex_array_struct *struct_a,
struct flex_array_struct *struct_b) {
*struct_b = *struct_a;
} |
When the structure is copied, the size of the flexible array member is not considered, and only the first member of the structure, num
, is copied, leaving the array contents untouched.
Compliant Solution (Copying)
This compliant solution uses memcpy()
to properly copy the content of struct_a
into struct_b
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <string.h>
struct flex_array_struct {
size_t num;
int data[];
};
void func(struct flex_array_struct *struct_a,
struct flex_array_struct *struct_b) {
if (struct_a->num > struct_b->num) {
/* Insufficient space; handle error */
return;
}
memcpy(struct_b, struct_a,
sizeof(struct flex_array_struct) + (sizeof(int)
* struct_a->num));
} |
Noncompliant Code Example (Function Arguments)
In this noncompliant code example, the flexible array structure is passed by value to a function that prints the array elements:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h>
#include <stdlib.h>
struct flex_array_struct {
size_t | ||||
Code Block | ||||
| ||||
struct flexArrayStruct{ int num; int data[]; }; /* ... */ void print_array(struct flex_array_struct struct_p) { puts("Array is: "); for (size_t i = 0; i < struct_p.num; ++i) { printf("%d ", struct_p.data[i]); } putchar('\n'); } void func(void) { struct flex_array_struct *struct_p; size_t array_size = 4; /* Space is allocated for the struct */ struct flexArrayStruct *structPstruct_p = (struct flexArrayStructflex_array_struct *)malloc( sizeof(struct flexArrayStruct) flex_array_struct) + sizeof(int) * ARRAYarray_SIZEsize); if (structPstruct_p == NULL) { /* handleHandle mallocerror failure */ } structP struct_p->num = SOMEarray_NUMBERsize; /* Access data[] as if it had been allocated as data[ARRAY_SIZE] */ for (for (size_t i = 0; i < ARRAYarray_SIZEsize; i++i) { structPstruct_p->data[i] = i; } |
Wiki Markup |
---|
This compliant solution allows the structure to be treated as if it had declared the member {{data\[\]}} to be {{data\[ARRAY_SIZE\]}} in a manner that conforms to the C99 standard. |
However, some restrictions apply:
- The incomplete array type must be the last element within the structure.
- There cannot be an array of structures that contain flexible array members.
- Structures that contain a flexible array member cannot be used as a member in the middle of another structure.
- The
sizeof
operator cannot be applied to a flexible array.
Risk Assessment
print_array(*struct_p);
} |
Because the argument is passed by value, the size of the flexible array member is not considered when the structure is copied, and only the first member of the structure, num
, is copied.
Compliant Solution (Function Arguments)
In this compliant solution, the structure is passed by reference and not by value:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h>
#include <stdlib.h>
struct flex_array_struct {
size_t num;
int data[];
};
void print_array(struct flex_array_struct *struct_p) {
puts("Array is: ");
for (size_t i = 0; i < struct_p->num; ++i) {
printf("%d ", struct_p->data[i]);
}
putchar('\n');
}
void func(void) {
struct flex_array_struct *struct_p;
size_t array_size = 4;
/* Space is allocated for the struct and initialized... */
print_array(struct_p);
} |
Risk Assessment
Failure to use structures with flexible array members correctly can result in undefined behavior. Failing to use the correct syntax can result in undefined behavior, although the (incorrect) syntax will work on most implementations.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM33-C | Low | Unlikely | Low | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Astrée |
| flexible-array-member-assignment flexible-array-member-declaration | Fully checked | ||||||
Axivion Bauhaus Suite |
| CertC-MEM33 | Fully implemented | ||||||
CodeSonar |
| LANG.STRUCT.DECL.FAM | Declaration of Flexible Array Member | ||||||
Compass/ROSE | Can detect all of these | ||||||||
Cppcheck Premium |
| premium-cert-mem33-c | Partially implemented | ||||||
Helix QAC |
| C1061, C1062, C1063, C1064 | |||||||
Klocwork |
| MISRA.INCOMPLETE.STRUCT | |||||||
LDRA tool suite |
| 649 S, 650 S | Fully implemented | ||||||
Parasoft C/C++test |
| CERT_C-MEM33-a | Allocate structures containing a flexible array member dynamically | ||||||
| CERT C: Rule MEM33-C |
1 (low)
1 (unlikely)
3 (low)
P3
Checks for misuse of structure with flexible array member (rule fully covered) | |||||||||
RuleChecker |
| flexible-array-member-assignment flexible-array-member-declaration | Fully checked |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Key here (explains table format and definitions)
Taxonomy | Taxonomy item | Relationship |
---|---|---|
CERT C Secure Coding Standard | DCL38-C. |
...
References
Wiki Markup |
---|
\[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] Section 6.7.2.1, "Structure and union specifiers"
\[[McCluskey 01|AA. C References#McCluskey 01]\] ;login:, July 2001, Volume 26, Number 4 |
Use the correct syntax when declaring a flexible array member | Prior to 2018-01-12: CERT: Unspecified Relationship |
CERT-CWE Mapping Notes
Key here for mapping notes
CWE-401 and MEM33-CPP
There is no longer a C++ rule for MEM33-CPP. (In fact, all C++ rules from 30-50 are gone, because we changed the numbering system to be 50-99 for C++ rules.)
Bibliography
[ISO/IEC 9899:2024] | Subclause 6.7.3.2, "Structure and Union Specifiers" |
[JTC1/SC22/WG14 N791] | Solving the Struct Hack Problem |
...
MEM32-C. Detect and handle critical memory allocation errors 08. Memory Management (MEM)