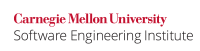
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\]: |
A nested class is any class whose declaration occurs within the body of another class or interface
...
Nested classes are a broad set of classes that are classified as static member and inner classes. "An inner class is a nested class that is not explicitly or implicitly declared {{static}}." \ [[JLS 05|AA. Java References#JLS 05]\]. An inner class may be local, anonymous or non-static.JLS 2015]. The use of a nested class is error - prone unless the semantics are well understood. A common notion is that only the outer nested class can may access the contents of the nested inner outer class(es). Not only does the nested class have access to the private fields of the outer class, but the same fields can be accessed by another any other class in within the package depending on whether when the nested class is declared public or if it contains public methods /or constructors. Wiki Markup Also, according to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\As a result, the nested class must not expose the private members of the outer class to external classes or packages. Wiki Markup
According to The Java Language Specification (JLS), §8.3, "Field Declarations" [JLS 2015]:
Note that a private field of a superclass might be accessible to a subclass (for example, if both classes are members of the same class). Nevertheless, a private field is never inherited by a subclass.
Noncompliant Code Example
The This noncompliant code in this noncompliant example illegally exposes the private (x,y)
coordinates through the getPoint()
method of the inner class. As a resultConsequently, the AnotherClass
class can illegally that belongs to the same package can also access the coordinates.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; public class Point { public void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } } |
Compliant Solution
Use the private
access specifier for declaring to hide the inner class (es) and all contained methods and constructors. The compiler will refuse to compile AnotherClass
because of its attempt to access a private nested class.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; private class Point { private void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); // failsFails to compile p.getPoint(); } } |
Compilation of AnotherClass
now results in a compilation error because the class attempts to access a private nested class.
Risk Assessment
The Java Language System language system weakens the access accessibility of private entities in inner classes which may result in a security weaknessmembers of an outer class when a nested inner class is present, which can result in an information leak.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
OBJ08-J |
Medium |
Probable |
Medium | P8 | L2 |
Automated Detection
...
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
...
Automated detection of nonprivate inner classes that define nonprivate members and constructors that leak private data from the outer class is straightforward.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.CLASS.ICSBS | Inner Class Should be Static (Java) | ||||||
Parasoft Jtest |
| CERT.OBJ08.INNER | Make all member classes "private" |
Related Guidelines
Bibliography
...
...
...
...
Section 2.3, "Inner Classes" | |
Securing Java: Getting Down to Business with Mobile Code |
...
Instances|http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.1.3] and 8.3 "Field Declarations" \[[McGraw 00|AA. Java References#McGraw 00]\] \[[Long 05|AA. Java References#Long 05]\] Section 2.3, Inner Classes \[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 492|http://cwe.mitre.org/data/definitions/492.html] "Use of Inner Class Containing Sensitive Data"SCP01-J. Do not increase the accessibility of overridden or hidden methods 04. Scope (SCP) SCP03-J. Do not reuse names