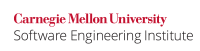
Methods can return values to signify communicate failure or success or , at other times, to update the caller's local objects or fields. Security risks can arise if when method return values are simply ignored or if suitable action is not taken on their receiptwhen the invoking method fails to take suitable action. Consequently, programs must not ignore method return values.
When getter methods are named after an action, a programmer could fail to realize that a return value is expected. For example, the only purpose of the ProcessBuilder.redirectErrorStream()
method is to report via return value whether the process builder successfully merged standard error and standard output. The method that actually performs redirection of the error stream is the overloaded single-argument method ProcessBuilder.redirectErrorStream(boolean)
.
Noncompliant Code Example (File Deletion)
This noncompliant code example attempts to delete a file , but does not fails to check that whether the operation succeeds.has succeeded:
Code Block | ||
---|---|---|
| ||
public void deleteFile(){ File someFile = new File("someFileName.txt"); // doDo something with someFile someFile.delete(); } |
Compliant Solution
In the This compliant solution , checks the (boolean
) Boolean value returned by the delete()
method is checked and, if necessary, the error is handled.and handles any resulting errors:
Code Block | ||
---|---|---|
| ||
public void deleteFile(){ File someFile = new File("someFileName.txt"); // doDo something with someFile if (!someFile.delete()) { // handleHandle thefailure factto thatdelete the file has not been deleted } } |
Noncompliant Code Example (String Replacement)
This noncompliant code example ignores the return value while making use of the String.replace()
method. As a result, , failing to update the original string is not updated even though it seems otherwise. The String.replace()
method does not cannot modify the state of the String
but instead, String
(because String
objects are immutable); rather, it returns a reference to a new String
object with the replacements in effectcontaining the modified string.
Code Block | ||
---|---|---|
| ||
public class IgnoreReplace { public static void main(String[] args) { String original = "insecure"; original.replace( 'i', '9' ); System.out.println(original); } } |
It is especially important to process the return values of immutable object methods. Although many methods of mutable objects operate by changing some internal state of the object, methods of immutable objects cannot change the object and often return a mutated new object, leaving the original object unchanged.
Compliant Solution
The This compliant solution correctly updates the original
string object by assigning to it String
reference original
with the return value from the String.replace()
method:
Code Block | ||
---|---|---|
| ||
public class DoNotIgnoreReplace { public static void main(String[] args) { String original = "insecure"; original = original.replace( 'i', '9' ); System.out.println(original); } } |
...
Risk Assessment
Ignoring method return values may can lead to erroneous computation which, in turn, may lead to vulnerabilitiesunexpected program behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
EXP00-J |
Medium |
Probable |
Medium | P8 | L2 |
Automated Detection
...
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.NULL.RET.UNCHECKED | Call Might Return Null (Java) | ||||||
Coverity | 7.5 | CHECKED_RETURN | Implemented | ||||||
Parasoft Jtest |
| CERT.EXP00.NASSIG CERT.EXP00.AECB | Ensure method and constructor return values are used Avoid "try", "catch" and "finally" blocks with empty bodies | ||||||
PVS-Studio |
| V6010, V6101 | |||||||
SonarQube |
| Return values from functions without side effects should not be ignored Return values should not be ignored when they contain the operation status code | |||||||
SpotBugs |
| RV_RETURN_VALUE_IGNORED | Implemented |
Related Guidelines
...
...
...
...
...
Passing Parameters and Return Values [CSJ] | |
CWE-252, Unchecked Return Value |
Bibliography
[API 2006] | |
Misusing | |
[Seacord 2015] |
...
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] method [delete()|http://java.sun.com/javase/6/docs/api/java/io/File.html#delete()]
\[[API 06|AA. Java References#API 06]\] method [replace()|http://java.sun.com/javase/6/docs/api/java/lang/String.html#replace(char,%20char)]
\[[Green 08|AA. Java References#Green 08]\] ["String.replace"|http://mindprod.com/jgloss/gotchas.html]
\[[Pugh 09|AA. Java References#Pugh 09]\] misusing putIfAbsent
\[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 252|http://cwe.mitre.org/data/definitions/252.html] "Unchecked Return Value" |
EXP01-J. Ensure a null pointer is not dereferenced 03. Expressions (EXP) EXP03-J. Do not compare String objects using equality or relational operators