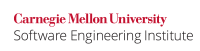
Member methods of non-final classes Nonfinal member methods that perform security checks can be compromised when a malicious subclass overrides the methods and omits the checks. Consequently, such methods must be declared private or final to prevent overriding.
...
This noncompliant code example allows a subclass to override the readSensitiveFile()
method and omit the required security check.:
Code Block | ||
---|---|---|
| ||
public void readSensitiveFile() {
try {
SecurityManager sm = System.getSecurityManager();
if (sm != null) { // Check for permission to read file
sm.checkRead("/temp/tempFile");
}
// Access the file
} catch (SecurityException se) {
// Log exception
}
}
|
...
This compliant solution prevents overriding of the readSensitiveFile()
method by declaring it final.:
Code Block | ||
---|---|---|
| ||
public final void readSensitiveFile() {
try {
SecurityManager sm = System.getSecurityManager();
if (sm != null) { // Check for permission to read file
sm.checkRead("/temp/tempFile");
}
// Access the file
} catch (SecurityException se) {
// Log exception
}
}
|
...
This compliant solution prevents overriding of the readSensitiveFile()
method by declaring it private.:
Code Block | ||
---|---|---|
| ||
private void readSensitiveFile() {
try {
SecurityManager sm = System.getSecurityManager();
if (sm != null) { // Check for permission to read file
sm.checkRead("/temp/tempFile");
}
// Access the file
} catch (SecurityException se) {
// Log exception
}
}
|
Exceptions
MET03-J-EX0: Classes that are declared final are exempt from this guideline rule because their member methods cannot be overridden.
Risk Assessment
Failure to declare a non-final class's method private or final affords the opportunity for a malicious subclass to bypass the security checks performed in the method.
Guideline Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET03-J | medium Medium | probable Probable | medium Medium | P8 | L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
Wiki Markup |
---|
\[[Ware 2008|AA. Bibliography#Ware 08]\] |
Android Implementation Details
On Android, System.getSecurityManager()
is not used, and the use of a security manager is not exercised. However, an Android developer can implement security-sensitive methods, so the principle may be applicable on Android.
Bibliography
IH.2.b.b. Declare methods that enforce |
...
MET02-J. Never use assertions to validate method parameters 05. Methods (MET) MET04-J. Ensure that constructors do not call overridable methods