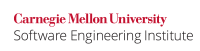
The enhanced for
statement introduced in Java 1.5, commonly referred to as the for-each idiom, is primarily used for iterating over collections of objects. While similar to the for
statement, this idiom cannot be used to assign values is designed for iteration through Collections and arrays.
The Java Language Specification (JLS) provides the following example of the enhanced for
statement in §14.14.2, "The Enhanced for
Statement" [JLS 2014]:
The enhanced for statement is equivalent to a basic for statement of the form:
Code Block for (I #i = Expression.iterator(); #i.hasNext(); ) { {VariableModifier} TargetType Identifier = (TargetType) #i.next(); Statement }
#i is an automatically generated identifier that is distinct from any other identifiers (automatically generated or otherwise) that are in scope...at the point where the enhanced for statement occurs.
Unlike the basic for
statement, assignments to the loop variable fail to affect the loop's iteration order over the underlying set of objects. Consequently, an assignment to the loop variable is equivalent to modifying a variable local to the loop body whose initial value is the object referenced by the loop iterator. This modification is not necessarily erroneous but can obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for
statement.
Declare all enhanced for
statement loop variables final. The final
declaration causes Java compilers to flag and reject any assignments made to the loop variable.
Noncompliant Code Example
This noncompliant code example attempts to initialize a Character
array process a collection of integers using an enhanced for
loop. However, because the loop variable cannot be assigned to, the array is not suitably initialized.It further intends to modify one item in the collection for processing:
Code Block | |||||
---|---|---|---|---|---|
| |||||
List<Integer> list Character[] array = Arrays.asList(new CharacterInteger[10]; for(Character c: array) c = 'x'; // initialization attempt for(int i=0;i<array.length;i++) ] {13, 14, 15}); boolean first = true; System.out.println("Processing list..."); for (Integer i: list) { if (first) { first = false; i = new Integer(99); } System.out.println(" New item: " + i); // Process i } System.out.println("Modified list?"); for (Integer i: list) { System.out.print(array[i]); // prints 10 "null"sprintln("List item: " + i); } |
However, this code does not actually modify the list, as shown by the program's output:
Processing list...
New item: 99
New item: 14
New item: 15
Modified list?
List item: 13
List item: 14
List item: 15
Compliant Solution
Declaring i
to be final mitigates this problem by causing the compiler to fail to permit i
to be assigned a new value:
Code Block | ||||
---|---|---|---|---|
| ||||
// ...
for (final Integer i: list) {
// ...
|
Compliant Solution
This compliant solution correctly initializes the array using a for loop.processes the "modified" list but leaves the actual list unchanged:
Code Block | ||||
---|---|---|---|---|
| ||||
Character[] array = new Character[10];
for(int i=0;i<array.length;i++)
array[i] = 'x';
|
Risk Assessment
// ...
for (final Integer i: list) {
Integer item = i;
if (first) {
first = false;
item = new Integer(99);
}
System.out.println(" New item: " + item);
// Process item
}
// ... |
Risk Assessment
Assignments to the loop variable of an Attempts to assign to the loop variable from within the enhanced for
loop (for-each
idiom) are futile and may leave the class fail to affect the overall iteration order, lead to programmer confusion, and can leave data in a fragile , or inconsistent state.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
DCL02-J |
Low |
Unlikely |
Low | P3 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
TODO
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] Section [14.14.2|http://java.sun.com/docs/books/jls/third_edition/html/statements.html#14.14.2] "The enhanced for statement" |
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Parasoft Jtest |
| CERT.DCL02.ITMOD | Do not modify collection while iterating over it |
Bibliography
...
DCL06-J. Beware integer literals beginning with '0'. 01. Declarations and Initialization (DCL) 01. Declarations and Initialization (DCL)