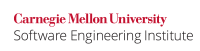
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\], section 8.4.8.3 "Requirements in Overriding and Hiding":Increasing the accessibility of overridden or hidden methods permits a malicious subclass to offer wider access to the restricted method than was originally intended. Consequently, programs must override methods only when necessary and must declare methods final whenever possible to prevent malicious subclassing. When methods cannot be declared final, programs must refrain from increasing the accessibility of overridden methods. Wiki Markup
The access modifier of an overriding or hiding method must provide at least as much access as the overridden or hidden method
...
(The Java Language Specification, §8.4.8.3, "Requirements in Overriding and Hiding" [JLS 2015]). The following table lists the allowed accesses.
Overridden/Hidden Method Modifier | Overriding/Hiding Method Modifier |
---|
The allowed accesses are:
Overridden/hidden method modifier
|
|
|
|
default | default or |
| Cannot be overridden |
This means that there is potential for some functionality having a restrictive access modifier to be overridden by a less restrictive access modifier.
Noncompliant Code Example
This noncompliant code example exemplifies demonstrates how a malicious subclass Sub
can both override the doLogic()
method of the superclass and increase the accessibility of the overriding method. Any user of Sub
will be able to can invoke the doLogic
method as because the base class BadScope
Super
defines it with the protected
access modifier. The class Sub
can allow more access than BadScope
to be protected
, consequently allowing class Sub
to increase the accessibility of doLogic()
by declaring its own version of the doLogic()
method {{public}method to be public.
Code Block | ||
---|---|---|
| ||
class BadScopeSuper { protected void doLogic() { System.out.println("Super invoked"); } } public class Sub extends BadScopeSuper { public void doLogic() { System.out.println("Sub invoked"); // Do sensitive operations } } |
Compliant Solution
Do not override a method unless absolutely necessary. Declare all methods and fields final
to avoid malicious subclassing. When this is not possible, refrain from increasing the accessibility of overridden methods. This is in compliance with the tenets of SEC01-J. Minimize the accessibility of classes and their members.This compliant solution declares the doLogic()
method final to prevent malicious overriding:
Code Block | ||
---|---|---|
| ||
class BadScopeSuper { protected final void doLogic() { // declareDeclare as final System.out.println("Super invoked"); // Do sensitive operations } } |
Noncompliant Code Example
This noncompliant code example overrides the finalize()
method of the superclass Base
, and changes its accessibility from protected
to public
.
According to Sun's Secure Coding Guidelines [[SCG 07]]:
In addition, refrain from increasing the accessibility of an inherited method, as doing so may break assumptions made by the superclass. A class that overrides the
protected java.lang.Object.finalize
method and declares that methodpublic
, for example, enables hostile callers to finalize an instance of that class, and to call methods on that instance after it has been finalized. A superclass implementation unprepared to handle such a call sequence could throw runtime exceptions that leak private information, or that leave the object in an invalid state that compromises security.
Code Block | ||
---|---|---|
| ||
final class SubClass extends Base {
public void finalize() {
// ...
}
}
|
Compliant Solution
This compliant solution correctly declares the finalize()
method protected
. It is not possible to further limit the accessibility as Object
's finalize
method itself is declared protected
.
Code Block | ||
---|---|---|
| ||
final class SubClass extends Base {
protected void finalize() {
// ...
}
}
|
It is recommended but not mandatory to limit the accessibility of a subclass's constructor to that of the superclass's constructor.
Exceptions
EX1: According to Sun's Secure Coding Guidelines [[SCG 07]]:
...
Exceptions
MET04-J-EX0: For classes that implement the java.lang.Cloneable
interface
...
, the accessibility of the Object.clone()
method should be increased from protected
to public
[SCG 2009].
Risk Assessment
Subclassing allows weakening of access restrictions to be weakened, possibly compromising , which can compromise the security of a Java application.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
MET04-J |
Medium |
Probable |
Medium | P8 | L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] [Section 8.4.8.3, Requirements in Overriding and Hiding|http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.4.8.3]
[\[[SCG 07|AA. Java References#SCG 07]\]] Guideline 1-1 Limit the accessibility of classes, interfaces, methods, and fields
\[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 487|http://cwe.mitre.org/data/definitions/487.html] "Reliance on Package-level Scope" |
Detecting violations of this rule is straightforward.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Parasoft Jtest |
| CERT.MET04.OPM | Do not override an instance "private" method |
Related Guidelines
CWE-487, Reliance on Package-Level Scope | |
Guideline 4-1 / EXTEND-1: Limit the accessibility of classes, interfaces, methods, and fields |
Bibliography
...
SCP00-J. Use as minimal scope as possible for all variables 05. Scope (SCP) SCP02-J. Do not reuse names