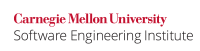
Note | ||
---|---|---|
| ||
This guideline has been deprecated. It has been merged with: 06/15/2015 -- Version 1.0 |
Similarly, a final
method parameter obtains an immutable copy of the object reference. Again, this has no effect on the mutability of the referenced data.
Noncompliant Code Example (Mutable Class, final
Reference)
In this noncompliant code example, the programmer has declared the reference to the point
instance to be final
under the incorrect assumption that doing so prevents modification of the values of the instance fields x
and y
. The values of the instance fields can be changed after their initialization because the final
clause applies only to the reference to the point
instance and not to the referenced object
Sometimes, when a variable is declared final
, it is believed to be immutable. If the variable is a primitive type, declaring it final
means that its value cannot be subsequently changed. However, if the variable is a reference to a mutable object, the object's contained data that appears to be immutable may actually be mutable. Consider for example, a final
method parameter that is a reference to an object. The argument to this method will use pass-by-value to copy the reference but the referenced data will remain mutable.
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\], section 4.12.4 "{{final}} Variables": |
... if a final variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
Noncompliant Code Example
In this example, the values of instance fields a
and b
can be changed even after their initialization. When an object reference is declared final
, it only signifies that the reference cannot be changed, whereas the contents that it refers to can still be.
Code Block | ||
---|---|---|
| ||
class Point FinalClass{ private int ax; private int by; FinalClassPoint(int ax, int by) { this.ax = ax; this.by = by; } void set_abxy(int ax, int by) { this.ax = ax; this.by = by; } void print_abxy() { System.out.println("the value ax is: " + this.ax); System.out.println("the value by is: " + this.by); } } public class FinalCallerPointCaller { public static void main(String[] args) { final FinalClassPoint fcpoint = new FinalClassPoint(1, 2); fcpoint.print_abxy(); //now weChange change the value of ax,b. y fcpoint.set_abxy(5, 6); fcpoint.print_abxy(); } } |
Compliant Solution
...
(final
Fields)
When the values of the x
and y
instance variables must remain If a
and b
have to be kept immutable after their initialization, the simplest approach is to declare them as final
.they should be declared final
. However, this invalidates a set_xy()
method because it can no longer change the values of x
and y
:
Code Block | ||
---|---|---|
| ||
class Point { private final int ax; private final int by; void set_abPoint(int ax, int by) { //But now the compilerthis.x complains= about set_ab method x; this.ay = ay; } void print_xy() { System.out.println("the value x is: " + this.b = b; } |
The problem with this method is that setter methods cannot be used to alter a
and b
.
Compliant Solution
.x);
System.out.println("the value y is: " + this.y);
}
// set_xy(int x, int y) no longer possible
}
|
With this modification, the values of the instance variables become immutable and consequently match the programmer's intended usage model.
Compliant Solution (Provide Copy Functionality)
If the class must remain mutable, another compliant solution is to provide copy functionality. This compliant solution provides a clone()
method in the class Point
, avoiding the elimination of the setter method:An alternative approach is to provide a clone
method in the class. The clone method can be used to get a copy of the original object. This new object can be freely used without affecting the original object.
Code Block | ||
---|---|---|
| ||
final public class NewFinalPoint implements Cloneable { private int ax; private int y; Point(int x, int b; NewFinal(int a y) { this.x = x; this.y = y; } void set_xy(int x, int by) { this.ax = ax; this.by = by; } void print_abxy() { System.out.println("the value ax is: "+ this.ax); System.out.println("the value by is: "+ this.by); } public voidPoint set_abclone(int) a, int b)throws CloneNotSupportedException{ this.aPoint cloned = a; this.b = b(Point) super.clone(); } public// NewFinalNo clone()need throwsto CloneNotSupportedException{ clone x and y NewFinalas clonedthey = (NewFinal) super.clone();are primitives return cloned; } } public class NewFinalCallerPointCaller { public static void main(String[] args) throws CloneNotSupportedException { finalPoint NewFinal nfpoint = new NewFinalPoint(1, 2); // Is not changed in main() nfpoint.print_abxy(); //get Get the copy of original object Point pointCopy try { NewFinal nf2 = nf.clone(); // change= point.clone(); // pointCopy now holds a unique reference to the // newly cloned Point instance // Change the value of ax,by of the copy. nf2pointCopy.set_abxy(5, 6); // originalOriginal value will not be changedremains unchanged nfpoint.print_abxy(); } catch (CloneNotSupportedException e) { /* Forward to handler */ } } } |
The class is made final to prevent subclasses from overriding the clone()
method . This enables the class to be accessed and used, while preventing the fields from being modified, and complies with OBJ36returns a copy of the original object that reflects the state of the original object at the moment of cloning. This new object can be used without exposing the original object. Because the caller holds the only reference to the newly cloned instance, the instance fields cannot be changed without the caller's cooperation. This use of the clone()
method allows the class to remain securely mutable. (See OBJ04-J. Provide mutable classes with a clone method copy functionality to safely allow passing instances to untrusted code safely.
Noncompliant Code Example
Another common mistake is to use a public static final
array. Clients can trivially modify the contents of the array (although they will not be able to change the array itself, as it is final).
Wiki Markup |
---|
With this declaration, {{SOMETHINGS\[1\]}}, etc. can be modified by clients of the code. |
Code Block | ||
---|---|---|
| ||
public static final SomeType [] SOMETHINGS = { ... };
|
Compliant Solution
One approach is to make use of the above method: first define a private
array and then provide a public
method that returns a copy of the array.
Code Block | ||
---|---|---|
| ||
private static final SomeType [] SOMETHINGS = { ... };
public static final SomeType [] somethings() {
return SOMETHINGS.clone();
}
|
Now the original array values cannot be modified by a client.
Compliant Solution
An alternative approach is to have a private
array from which a public
immutable list is constructed:
Code Block | ||
---|---|---|
| ||
private static final SomeType [] THE_THINGS = { ... };
public static final List<SomeType> SOMETHINGS =
Collections.unmodifiableList(Arrays.asList(THE_THINGS));
|
Now neither the original array values nor the public
list can be modified by any client.
Risk Assessment
Using final
to declare the reference to a mutable object is a potential security risk because the contents of the object can still be changed.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ03- J | low | probable | medium | P4 | L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] Sections 4.12.4 "final Variables" and 6.6, "Access Control"
\[[Bloch 08|AA. Java References#Bloch 08]\] Item 13: Minimize the accessibility of classes and members
\[[Core Java 04|AA. Java References#Core Java 04]\] Chapter 6
\[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 607|http://cwe.mitre.org/data/definitions/607.html] "Public Static Final Field References Mutable Object" |
)
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object.
Applicability
Incorrectly assuming that final
references cause the contents of the referenced object to remain mutable can result in an attacker modifying an object believed to be immutable.
Bibliography
Item 13, "Minimize the Accessibility of Classes and Members" | |
Chapter 6, "Interfaces and Inner Classes" | |
[JLS 2013] |
...
OBJ02-J. Avoid using finalizers 07. Object Orientation (OBJ) OBJ04-J. Encapsulate the absence of an object by using a Null Object