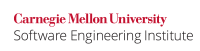
The enhanced for
statement introduced in Java V1.5 (also known as the for-each idiom) is primarily used for iterating over collections of objects. Unlike the original for
statement, assignments to the loop variable fail to affect the loop's iteration order over the underlying set of objects. Consequently, assignments to the loop variable can have an effect other than what is intended by the developer. This provides yet another reason to avoid assigning to the loop variable in a for
loop.
Wiki Markup |
---|
As detailed in [§14.14.2, "The Enhanced For Statement" |http://java.sun.com/docs/books/jls/third_edition/html/statements.html#14.14.2] of the _Java Language Specification_ \[[JLS 2005|AA. Bibliography#JLS 05]\] |
is designed for iteration through Collections and arrays.
The Java Language Specification (JLS) provides the following example of the enhanced for
statement in §14.14.2, "The Enhanced for
Statement" [JLS 2014]:
The enhanced for statement is equivalent to a basic for statement of the form:
Code Block for (I #i = Expression
an enhanced
for
statement of the form
Code Block for (ObjType obj : someIterableItem) { // ... }
is equivalent to a standard
for
loop of the form
Code Block for (Iterator myIterator = someIterableItem.iterator(); iterator#i.hasNext(); ) { {VariableModifier} ObjTypeTargetType objIdentifier = myIterator (TargetType) #i.next(); // ... } Statement }#i is an automatically generated identifier that is distinct from any other identifiers (automatically generated or otherwise) that are in scope...at the point where the enhanced for statement occurs.
Unlike the basic for
statement, assignments to the loop variable fail to affect the loop's iteration order over the underlying set of objects. Consequently, an assignment to the loop variable is equivalent to modifying a variable local to the loop body whose initial value is the object referenced by the loop iterator. This modification is not necessarily erroneous , but it can obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for
statement.
Declare all enhanced for
statement loop variables to be final. The final
declaration causes Java compilers to flag and reject any assignments made to the loop variable.
Noncompliant Code Example
This noncompliant code example attempts to process a collection of objects integers using an enhanced for
loop. It further intends to skip processing modify one item in the collection .for processing:
Code Block | |||||
---|---|---|---|---|---|
| |||||
List<Integer> list = Arrays.asList(new Integer[] {13, 14, 15}); boolean first = true; System.out.println("Processing list..."); Collection<ProcessObj> processThese = // ... for (ProcessObjInteger processMei: processTheselist) { if (someConditionfirst) { // found the itemfirst to= skipfalse; someConditioni = false;new Integer(99); } System.out.println(" New processMe = processMe.getNext(item: " + i); // attempt to skip to next item } processMe.doTheProcessing(); // process the object } |
The attempt to skip to the next item is "successful" because the assignment is successful, and the value of processMe
is updated. Unlike an original for
loop, however, the assignment leaves the overall iteration order of the loop unchanged. As a result, the object following the skipped object is processed twice; this is unlikely to be what the programmer intended.
...
Process i
}
System.out.println("Modified list?");
for (Integer i: list) {
System.out.println("List item: " + i);
}
|
However, this code does not actually modify the list, as shown by the program's output:
Processing list...
New item: 99
New item: 14
New item: 15
Modified list?
List item: 13
List item: 14
List item: 15
Compliant Solution
Declaring i
to be final mitigates this problem by causing the compiler to fail to permit i
to be assigned a new value:
Code Block | ||||
---|---|---|---|---|
| ||||
// ...
for (final Integer i: list) {
// ...
|
Compliant Solution
This compliant solution correctly processes the objects in the collection not more than one time."modified" list but leaves the actual list unchanged:
Code Block | ||||
---|---|---|---|---|
| ||||
Collection<ProcessObj> processThese = // ... for (final ProcessObjInteger processMei: processTheselist) { ifInteger (someCondition)item { // found the item to skip= i; if (first) { someConditionfirst = false; continue;item //= skip by continuing to next iterationnew Integer(99); } processMeSystem.out.doTheProcessing(println(" New item: " + item); // processProcess the objectitem } // ... |
Risk Assessment
Assignments to the loop variable of an enhanced for
loop (for-each
idiom) fail to affect the overall iteration order, lead to programmer confusion, and can leave data in a fragile or inconsistent state.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
DCL02-J |
Low |
Unlikely |
Low | P3 | L3 |
Automated Detection
...
This rule is easily enforced with static analysis.
Bibliography
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Parasoft Jtest |
| CERT.DCL02.ITMOD | Do not modify collection while iterating over it |
Bibliography
...
enhanced for statement"|http://java.sun.com/docs/books/jls/third_edition/html/statements.html#14.14.2] 01. Declarations and Initialization (DCL) 01. Declarations and Initialization (DCL) DCL01-J. Avoid ambiguous overloading of varargs methods